- Joined
- Jul 18, 2010
- Messages
- 2,384
Introduction
TEXTAREA is a frame to display scrollable text. Normaly TextAreas have a BACKDROP showing a box and a Background.
TextAreas require a scrollbar. The scrollbar is at the right side of the TextArea and blocks a part of the space given to the TextArea. If the TextArea is big enough to display the whole text, the scrollbar is not shown. Even when the scrollbar is not shown it takes its space. For not scrollable Text one could simply use a TEXT-Frame.
TextAreas require a scrollbar. The scrollbar is at the right side of the TextArea and blocks a part of the space given to the TextArea. If the TextArea is big enough to display the whole text, the scrollbar is not shown. Even when the scrollbar is not shown it takes its space. For not scrollable Text one could simply use a TEXT-Frame.
Built in TextArea
Warcraft 3 has 2 builtIn TEXTAREA mainframes.
BattleNetTextAreaTemplate
EscMenuTextAreaTemplate
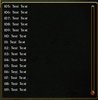
They are a good base to inherit, copy or one can just create them. If one wants to create one of them one would have to load them first using BlzLoadTOCFile.
The fdfs containing them are located at:
UI\Frames\framedef\glue\battlenettemplates.fdf
UI\Frames\framedef\ui\escmenutemplates.fdf
BattleNetTextAreaTemplate
EscMenuTextAreaTemplate
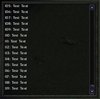
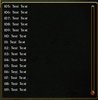
They are a good base to inherit, copy or one can just create them. If one wants to create one of them one would have to load them first using BlzLoadTOCFile.
The fdfs containing them are located at:
UI\Frames\framedef\glue\battlenettemplates.fdf
UI\Frames\framedef\ui\escmenutemplates.fdf
Creating builtin TextArea
This jass Code creates both TextAreas set the poistions, the size and fills them with text. It is quite important to give a TEXTAREA a size being big enough to display the scrollbar otherwise the game crashs on the attempt to display the TEXTAREA. The Height of the TextArea should be atleast 0.03. That is quite small, but if one forgets to set the size without having a default size, it will be 0 and the game crashs.
JASS:
function CreateDefaultTextAreas takes nothing returns nothing
local framehandle textArea = BlzCreateFrame("BattleNetTextAreaTemplate", BlzGetOriginFrame(ORIGIN_FRAME_GAME_UI, 0),0,0)
local framehandle textArea2 = BlzCreateFrame("EscMenuTextAreaTemplate", BlzGetOriginFrame(ORIGIN_FRAME_GAME_UI, 0),0,0)
local integer index = 1
call BlzFrameSetAbsPoint(textArea, FRAMEPOINT_TOPLEFT, 0.1, 0.5)
call BlzFrameSetAbsPoint(textArea2, FRAMEPOINT_TOPLEFT, 0.5, 0.5)
call BlzFrameSetSize(textArea, 0.25, 0.25)
call BlzFrameSetSize(textArea2, 0.25, 0.25)
loop
exitwhen index == 120
call BlzFrameAddText(textArea, I2S(index)+": Test Text")
call BlzFrameAddText(textArea2, I2S(index)+": Test Text")
set index = index + 1
endloop
endfunction
//===========================================================================
function InitTrig_Create_TextAreaTemplates takes nothing returns nothing
call BlzLoadTOCFile( "war3mapImported\\Templates.toc" )
call TimerStart(CreateTimer(), 0.0, false, function CreateDefaultTextAreas)
endfunction
Seting text of a TextArea
To set the text of a textarea one uses
For a TextArea BlzFrameGetText seems to be quite fugitive, after 1 second it returned null, while it was returning the total text in the same function as the text was set. I didn't tested this much.
TextArea can display colored Text using warcraft colorformat |cffff0000.
BlzFrameAddText(textArea, newLine)
or BlzFrameSetText(textArea, totalText)
. BlzFrameAddText adds a line Seperator before the new added text, if the TextArea already contained text.For a TextArea BlzFrameGetText seems to be quite fugitive, after 1 second it returned null, while it was returning the total text in the same function as the text was set. I didn't tested this much.
TextArea can display colored Text using warcraft colorformat |cffff0000.
Screen-Space and Scrolling
On Default a TextArea will catch the screen-space given to it, making it unclickable for the playable world and sending mousewhell rolls to its scrollbar. One could disable the TextArea to stop the TextArea from taking the mouse Input which would make the screenspace useable by the playable game again, but that would also disable the mouse whell roll sending. One could still scroll when the mouse points directly to the scrollbar.
Disabling is done with:
Disabling is done with:
call BlzFrameSetEnable(textArea, false)
TEXTAREA-FDF
This is a custom TEXTAREA named MyTextArea which has some comments describing the result of fields of TEXTAREA inside fdf:
TEXTAREA rejects most TEXT font Settings one might know when one used TEXT-Frame. Hence the text appereance can't be changed much.
jass Code creating MyTextArea from above.
TEXTAREA rejects most TEXT font Settings one might know when one used TEXT-Frame. Hence the text appereance can't be changed much.
Code:
IncludeFile "UI\FrameDef\UI\EscMenuTemplates.fdf", //Frames from that fdf can be inherited in this file
IncludeFile "UI\FrameDef\UI\QuestDialog.fdf",
Frame "TEXTAREA" "MyTextArea" {
DecorateFileNames,
FrameFont "InfoPanelTextFont", 0.020, "",
TextAreaLineHeight 0.018, //Should fit used font Size, a high difference shows no text or much space between lines.
TextAreaLineGap 0.00, //adds that much space between 2 Lines, addtional to TextAreaLineHeight.
TextAreaInset 0.035, //Moves the Text and the scrollbar into the middle from all sides by this amount. This increases the min size for game crash.
TextAreaMaxLines 20, //Only that amount of lines are displayed, if more lines are added oldest are removed.
TextAreaScrollBar "MyTextAreaScrollBar",
Frame "SCROLLBAR" "MyTextAreaScrollBar" INHERITS WITHCHILDREN "EscMenuScrollBarTemplate" {
}
ControlBackdrop "MyTextAreaBackdrop",
Frame "BACKDROP" "MyTextAreaBackdrop" INHERITS "QuestButtonBaseTemplate" {
}
}
jass Code creating MyTextArea from above.
JASS:
function CreateMyTextArea takes nothing returns nothing
local framehandle textArea = BlzCreateFrame("MyTextArea", BlzGetOriginFrame(ORIGIN_FRAME_GAME_UI, 0),0,0)
local integer index = 1
call BlzFrameSetAbsPoint(textArea, FRAMEPOINT_CENTER, 0.4, 0.3)
call BlzFrameSetSize(textArea, 0.25, 0.25)
loop
exitwhen index == 120
call BlzFrameAddText(textArea, I2S(index)+": Test Text")
set index = index + 1
endloop
endfunction
//===========================================================================
function InitTrig_Create_MyTextArea takes nothing returns nothing
call BlzLoadTOCFile( "war3mapImported\\MyTextArea.toc" )
call TimerStart(CreateTimer(), 0.0, false, function CreateMyTextArea)
endfunction
Inherited TextArea
If one is overall fine with a TextArea and only wants some small changes, like a different textsize. Then one could inherit from the wanted TextArea and mention only the changed textsize. That way one can have small (in definition) , powerful and still valid frames.
That could look like this
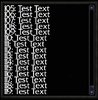
"BigBnetTextArea" is basicly "BattleNetTextAreaTemplate" but has a bigger TextSize and the lines are bigger, to fit better with the increased textsize.
To inherit a Frame's childFrames, one adds WITHCHILDREN after INHERITS.
BlzGetFrameByName is not able to return inherited childFrames. With the release of the native BlzFrameGetChild in V1.32.6, one is able to get the inherited childFrames during the runtime, in 1.32.6 this native ignores String&Texture childFrames.
That could look like this
Code:
IncludeFile "UI\FrameDef\glue\battlenettemplates.fdf", //Frames from that fdf can be inherited in this file
Frame "TEXTAREA" "BigBnetTextArea" INHERITS WITHCHILDREN "BattleNetTextAreaTemplate" {
DecorateFileNames,
FrameFont "MasterFont", 0.020, "",
TextAreaLineHeight 0.015,
}
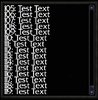
"BigBnetTextArea" is basicly "BattleNetTextAreaTemplate" but has a bigger TextSize and the lines are bigger, to fit better with the increased textsize.
To inherit a Frame's childFrames, one adds WITHCHILDREN after INHERITS.
BlzGetFrameByName is not able to return inherited childFrames. With the release of the native BlzFrameGetChild in V1.32.6, one is able to get the inherited childFrames during the runtime, in 1.32.6 this native ignores String&Texture childFrames.
Other UI-Frame Tutorials
The following links might provide more insight into this subject.
UI: Change Lumber Text
[JASS/AI] - UI: Create a TextButton
[JASS/AI] - UI: Positionate Frames (important)
UI: toc-Files
UI: Reading a FDF
UI - The concept of Parent-Frames
[JASS/AI] - UI: FrameEvents and FrameTypes
UI: Frames and Tooltips
[JASS/AI] - UI: Creating a Bar
UI - Simpleframes
UI: What are BACKDROPs?
UI: GLUEBUTTON
UI: TEXTAREA the scrolling Text Frame
UI: EditBox - Text Input
[JASS/AI] - UI: Creating a Cam control
UI: Showing 3 Multiboards
UI: OriginFrames
Default Names for BlzGetFrameByName (Access to Existing game-Frames)
[JASS/AI] - UI: List - Default MainFrames (built in CreateAble)
UI: Change Lumber Text
[JASS/AI] - UI: Create a TextButton
[JASS/AI] - UI: Positionate Frames (important)
UI: toc-Files
UI: Reading a FDF
UI - The concept of Parent-Frames
[JASS/AI] - UI: FrameEvents and FrameTypes
UI: Frames and Tooltips
[JASS/AI] - UI: Creating a Bar
UI - Simpleframes
UI: What are BACKDROPs?
UI: GLUEBUTTON
UI: TEXTAREA the scrolling Text Frame
UI: EditBox - Text Input
[JASS/AI] - UI: Creating a Cam control
UI: Showing 3 Multiboards
UI: OriginFrames
Default Names for BlzGetFrameByName (Access to Existing game-Frames)
[JASS/AI] - UI: List - Default MainFrames (built in CreateAble)
Attachments
Last edited by a moderator: