- Joined
- Jul 18, 2010
- Messages
- 2,379
Introduction
Frames positions are important and are the key to place frames where one wants them to be.
To positionate frames there exist 4 natives. All 4 have great uses.
To positionate frames one also needs the framepointtype constants.
To positionate frames there exist 4 natives. All 4 have great uses.
JASS:
native BlzFrameSetPoint takes framehandle frame, framepointtype point, framehandle relative, framepointtype relativePoint, real x, real y returns nothing
native BlzFrameSetAbsPoint takes framehandle frame, framepointtype point, real x, real y returns nothing
native BlzFrameClearAllPoints takes framehandle frame returns nothing
native BlzFrameSetAllPoints takes framehandle frame, framehandle relative returns nothing
JASS:
FRAMEPOINT_TOPLEFT
FRAMEPOINT_TOP
FRAMEPOINT_TOPRIGHT
FRAMEPOINT_LEFT
FRAMEPOINT_CENTER
FRAMEPOINT_RIGHT
FRAMEPOINT_BOTTOMLEFT
FRAMEPOINT_BOTTOM
FRAMEPOINT_BOTTOMRIGHT
What the natives do?
BlzFrameSetAbsPoint
takes one point of a Frame unbound that point and places it to a specific coordinates on the screen.BlzFrameSetPoint
places a point of FrameA relative to a point of FrameB. When FrameB moves FrameA's point will keep this rule and moves with it. The x/y-Offset Values are affected by FrameA Scale.BlzFrameClearAllPoints
removes all curent bound points of that frame.BlzFrameSetAllPoints
FrameA will copy FrameB in size and position. FrameA will update when FrameB is changed later.
Cartesian coordinate system
coordinates and offset are both a factor of the total screen. 0.0/0.0 beeing the bottom left corner of the 4:3 screen. 0.8/0.6 is the top right corner of the 4:3 screen.
Positionating requiers the frame one wants to move and the FRAMEPOINT of that frame. One moves only the choosen FRAMEPOINT, if other FRAMEPOINTS are still located somewhere, the frame will be stretched to have both FRAMEPOINTS be filled as good that is possible, for a rectangle. This can (sometimes has to) be used to define size of frames.
Here one should positionate either
When placing only one FramePoint the choosen FramePoint has a strong impact on the result. It limites the directions size/text can expand.
When using a point containing:
If one simply wants to move a frame having a size one should first use BlzFrameClearAllPoints to free already placed Points.
SetPoint requires the relative frame to take space on the screen, that relative frame can be set on a later time, but until that happens the moved frame will displayed wierdly or not all.
Positionating requiers the frame one wants to move and the FRAMEPOINT of that frame. One moves only the choosen FRAMEPOINT, if other FRAMEPOINTS are still located somewhere, the frame will be stretched to have both FRAMEPOINTS be filled as good that is possible, for a rectangle. This can (sometimes has to) be used to define size of frames.
Here one should positionate either
FRAMEPOINT_TOPRIGHT and FRAMEPOINT_BOTTOMLEFT
or FRAMEPOINT_TOPLEFT and FRAMEPOINT_BOTTOMRIGHT
of the frame one wants to set its size.When placing only one FramePoint the choosen FramePoint has a strong impact on the result. It limites the directions size/text can expand.
When using a point containing:
LEFT can only extend to the right x wise.
RIGHT can only extend to the left x wise.
CENTER extends equal in all directions
TOP can only extend downwards y wise
BOTTOM can only extend upwards y wise.
RIGHT can only extend to the left x wise.
CENTER extends equal in all directions
TOP can only extend downwards y wise
BOTTOM can only extend upwards y wise.
If one simply wants to move a frame having a size one should first use BlzFrameClearAllPoints to free already placed Points.
SetPoint requires the relative frame to take space on the screen, that relative frame can be set on a later time, but until that happens the moved frame will displayed wierdly or not all.
Relative Position
Frames that belong logical to some other Frame benefit much when one sets their position relative to that frame they belong to or relative to a frame that is relative to the owner that could be contiuned, but in the end it is relative to the Owner Frame. This relative position is updated when the other Frame is moved. Because of that one only needs to set the position of the not relative posed Frame in that group and everything moves with it. In code the native doing that is
Setting Relative position is a little bit inversed. when one was to place something left to something one needs to place the right side (of the moving) to the left of the target.
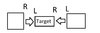
The x and y offset in BlzFrameSetPoint are added to the target Point. with +x the position anchored to is moved further to the right and with +y it is moved higher up. BlzFrameSetScale of the moving frame affect the x&y offset.
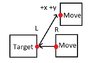
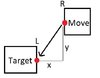
In Fdf one can only set position relative. But in Code one can also place them to a fixed position.
BlzFrameSetPoint
. Setting Relative position is a little bit inversed. when one was to place something left to something one needs to place the right side (of the moving) to the left of the target.
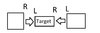
JASS:
BlzFrameSetPoint(frameLeft, FRAMEPOINT_RIGHT, frameTarget, FRAMEPOINT_LEFT, 0, 0)
BlzFrameSetPoint(frameRight, FRAMEPOINT_LEFT, frameTarget, FRAMEPOINT_RIGHT, 0, 0)
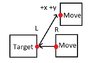
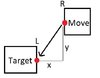
In Fdf one can only set position relative. But in Code one can also place them to a fixed position.
Example
Lets move the menu button somewhere else, somewhere to the right top corner.
Not what we wanted. The Menu button stretched far to the right, pushed the Allies & Log button to the right and did not move lower. It seems his left side is still connected to the Quest button.
It should work better, if we clear the points of Menu button.
Well the Menu button moved, also the Allies & Log buttons, the texts are bit off, but it moved away from the Quest button and is not stretched.
Lets move the Allies button below the Menu button and the Log button below the Allies button.
Menu Allies and Log in a col, text is where it should be, I like it.
They are to much in the center, but that is cause we moved menu with FRAMEPOINT_RIGHT. And cause of the wrong information of the total x size, the missinformation was 0.6 as max of the 4:3, but 0.8 is the right end of the 4:3 Screen not 0.6. 0.5 is much nearer to 0.4 then to 0.8 why it is more centered.
JASS:
function ReposMenuButtons takes nothing returns nothing
local framehandle fh = BlzGetFrameByName("UpperButtonBarMenuButton",0)
call BlzFrameSetAbsPoint(fh, FRAMEPOINT_RIGHT, 0.5, 0.5)
endfunction
Not what we wanted. The Menu button stretched far to the right, pushed the Allies & Log button to the right and did not move lower. It seems his left side is still connected to the Quest button.
It should work better, if we clear the points of Menu button.
JASS:
function ReposMenuButtons2 takes nothing returns nothing
local framehandle fh = BlzGetFrameByName("UpperButtonBarMenuButton",0)
call BlzFrameClearAllPoints(fh)
call BlzFrameSetAbsPoint(fh, FRAMEPOINT_RIGHT, 0.5, 0.5)
endfunction
Well the Menu button moved, also the Allies & Log buttons, the texts are bit off, but it moved away from the Quest button and is not stretched.
Lets move the Allies button below the Menu button and the Log button below the Allies button.
JASS:
function ReposMenuButtons3 takes nothing returns nothing
local framehandle allies = BlzGetFrameByName("UpperButtonBarAlliesButton",0)
local framehandle menu = BlzGetFrameByName("UpperButtonBarMenuButton",0)
local framehandle log = BlzGetFrameByName("UpperButtonBarChatButton",0)
call BlzFrameClearAllPoints(allies)
call BlzFrameClearAllPoints(log)
call BlzFrameClearAllPoints(menu)
call BlzFrameSetAbsPoint(menu, FRAMEPOINT_RIGHT, 0.5, 0.5) //Pos Menu
call BlzFrameSetPoint(allies, FRAMEPOINT_TOP, menu, FRAMEPOINT_BOTTOM, 0.0, 0.0) //Pos allies below menu
call BlzFrameSetPoint(log, FRAMEPOINT_TOP, allies, FRAMEPOINT_BOTTOM, 0.0, 0.0) //Pos log below allies
endfunction
Menu Allies and Log in a col, text is where it should be, I like it.
They are to much in the center, but that is cause we moved menu with FRAMEPOINT_RIGHT. And cause of the wrong information of the total x size, the missinformation was 0.6 as max of the 4:3, but 0.8 is the right end of the 4:3 Screen not 0.6. 0.5 is much nearer to 0.4 then to 0.8 why it is more centered.
Frame Pos in FDF
When one creates frames in fdf one can also set the position of frames. Here one has 3 options:
That Frame of type BACKDROP with Name Test displays, after it's loading & creation, a Paladin-Icon at the Top Left of the screen (Would have to be loaded over a TOC first).
SetAllPoints is equal to BlzFrameSetAllPoints(self, parent).
Anchor is BlzFrameSetPoint(self, point, parent, point, x, y).
SetPoint FramePoint-Own, FrameName, FramePoint-FrameName, xOffset , yOffset,
SetAllPoints,
Anchor FramePoint, x, y, (only Texture/String)
SetPoint can be seen as SetAllPoints,
Anchor FramePoint, x, y, (only Texture/String)
BlzFrameSetPoint(self, FramePoint-Own, BlzGetFrameByName(FrameName, 0), FramePoint-FrameName, x, y)
. With UseActiveContext, the Frame will connect to the relative Frame with the same CreateContext the current Frame is created with.
Code:
Frame "BACKDROP" "Test" {
Width 0.1,
Height 0.1,
SetPoint TOPLEFT, "ConsoleUI", TOPLEFT, 0, 0,
BackdropBackground "ReplaceableTextures\CommandButtons\BTNHeroPaladin",
}
SetAllPoints is equal to BlzFrameSetAllPoints(self, parent).
Anchor is BlzFrameSetPoint(self, point, parent, point, x, y).
Other UI-Frame Tutorials
The following links might provide more insight into this subject.
UI: Change Lumber Text
[JASS/AI] - UI: Create a TextButton
[JASS/AI] - UI: Positionate Frames (important)
UI: toc-Files
UI: Reading a FDF
UI - The concept of Parent-Frames
[JASS/AI] - UI: FrameEvents and FrameTypes
UI: Frames and Tooltips
[JASS/AI] - UI: Creating a Bar
UI - Simpleframes
UI: What are BACKDROPs?
UI: GLUEBUTTON
UI: TEXTAREA the scrolling Text Frame
UI: EditBox - Text Input
[JASS/AI] - UI: Creating a Cam control
UI: Showing 3 Multiboards
UI: OriginFrames
Default Names for BlzGetFrameByName (Access to Existing game-Frames)
[JASS/AI] - UI: List - Default MainFrames (built in CreateAble)
UI: Change Lumber Text
[JASS/AI] - UI: Create a TextButton
[JASS/AI] - UI: Positionate Frames (important)
UI: toc-Files
UI: Reading a FDF
UI - The concept of Parent-Frames
[JASS/AI] - UI: FrameEvents and FrameTypes
UI: Frames and Tooltips
[JASS/AI] - UI: Creating a Bar
UI - Simpleframes
UI: What are BACKDROPs?
UI: GLUEBUTTON
UI: TEXTAREA the scrolling Text Frame
UI: EditBox - Text Input
[JASS/AI] - UI: Creating a Cam control
UI: Showing 3 Multiboards
UI: OriginFrames
Default Names for BlzGetFrameByName (Access to Existing game-Frames)
[JASS/AI] - UI: List - Default MainFrames (built in CreateAble)
Attachments
Last edited: