- Joined
- Oct 10, 2013
- Messages
- 173
Hey there,
Lets cover up While loop a little in this tutorial with one example.
Things you need before reading this tutorial.
Things you will be able to do after the tutorial:
Lets start!
Meaning Of While Loop.
So first what is the meaning "of while"? Think then click spoiler.
Now tell me what is the meaning of "Loop in C"?Think then click spoiler.
Now merge them together, and now think the meaning of " While loop".
Now that should have cleared basic stand of meaning of while loop, lets go further.
Structure of While loop.
The structure of while loop is very simple.It looks like this.Do look the error section because of one common blunder!
while(condition)
{
Set of instructions(your desired functions)
}
Example of code using while loop.
Short simple code, the output of this code is given below in i
mage.
Working.
That's all folks.
Feedbacks are welcomed, trolls ignored. New learners are always welcomed.
Edit
The scan f error is more elobrated here, why actually the program crashes,
Here is an example
scanf("%d", &i);
will write an int data by the user to the memory location allocated for i.
If & is not placed before i, then scanf will try to write the input data to the memory location i instead of &i. Since i contains indeterminate value, there are some possibilities that it may contain the value equivalent to the value of a memory address or it may contain the value which is out of range of memory address.
Lets cover up While loop a little in this tutorial with one example.
Things you need before reading this tutorial.
- Must be familiar with scanf, and print f.
- Must have good knowledge about data types.
Things you will be able to do after the tutorial:
- You will be able to make some fun programs using While loop, and show off your friends.
- A bit concept clearance to some newcomers, I will be sharing my research here.
- Make some interest calculating program, using interesting logic's.
- Avoid some key errors, as I will guide your through my research.
Lets start!
Meaning Of While Loop.
So first what is the meaning "of while"? Think then click spoiler.
a period of time.
"we chatted for a while"
"we chatted for a while"
a loop is a sequence of instruction s that is continually repeated
A set of instructions repeating themselves, until certain condition is reached 
Structure of While loop.
The structure of while loop is very simple.It looks like this.Do look the error section because of one common blunder!
while(condition)
{
Set of instructions(your desired functions)
}
Example of code using while loop.
Code:
#include<stdio.h>
#include<conio.h>
void main()
{
int year,period;
float net,amount,rate;
printf("This is a simple program, which will tell you your salary in future considering all the raise.\n");
printf("Please enter your current salary\n");
scanf("%f", &amount);
printf("Now please enter the rate, at which you think your salary will increase\n");
scanf("%f",&rate);
printf("Enter the period, in which you want to calculate your salary hike\n");
scanf("%d",&period);
printf("Ok, so your salary is %f. Your desired rate is %f, and your time period is %d\n\n\n",amount,rate,period);
year=0;
while(year<=period)
{
year=year+1;
net=amount+amount*rate/100;
printf("Your salary at year %d is %f\n",year,net);
amount=net;
}
}
mage.
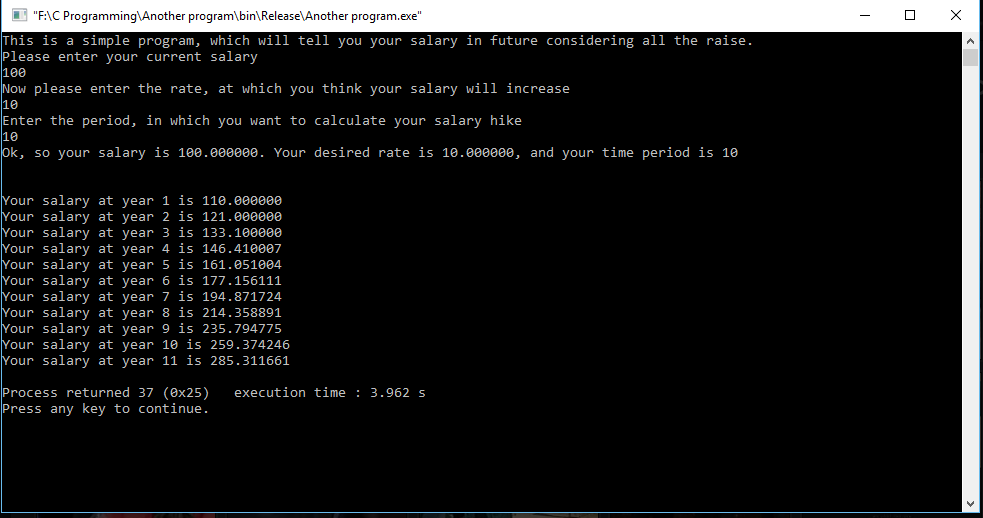
Working.
- The code above while loop has only one objective, that is to fetch data from user.
Code:#include<conio.h> void main() { int year,period; float net,amount,rate; printf("This is a simple program, which will tell you your salary in future considering all the raise.\n"); printf("Please enter your current salary\n"); scanf("%f", &amount); printf("Now please enter the rate, at which you think your salary will increase\n"); scanf("%f",&rate); printf("Enter the period, in which you want to calculate your salary hike\n"); scanf("%d",&period); printf("Ok, so your salary is %f. Your desired rate is %f, and your time period is %d\n\n\n",amount,rate,period);
- You would had noticed that the code
Code:
year=0;
- Now talking of while loop. Notice that the code structure mentioned matches in this code.
Code:while(year<=period) { year=year+1; net=amount+amount*rate/100; printf("Your salary at year %d is %f\n",year,net); amount=net; }
Code:(year<=period)
- These are the statements
Code:year=year+1; net=amount+amount*rate/100; printf("Your salary at year %d is %f\n",year,net); amount=net;
- Now you know that until the specific condition is reached this code will continue re executing its instruction inside the loop.Thus we put
Code:
year=year+1;
- Since our main algorithm to calculate interest is
Code:
net=amount+amount*rate/100;
Code:amount=net;
- The code above while loop has only one objective, that is to fetch data from user.
- If you skipped a "&" in scanf you will get this error.My advice to recheck the
- If you would had typed a ;(semi colon) after while(condition) like this while(condition); then the compiler think it is the end of code and won't go any further, or won't executes the code inside the while loop and will just skip through it.
- If you skipped a "&" in scanf you will get this error.My advice to recheck the
That's all folks.
Feedbacks are welcomed, trolls ignored. New learners are always welcomed.
Edit
The scan f error is more elobrated here, why actually the program crashes,
Here is an example
scanf("%d", &i);
will write an int data by the user to the memory location allocated for i.
If & is not placed before i, then scanf will try to write the input data to the memory location i instead of &i. Since i contains indeterminate value, there are some possibilities that it may contain the value equivalent to the value of a memory address or it may contain the value which is out of range of memory address.
Last edited: