- Joined
- Dec 6, 2006
- Messages
- 72
Tutorial: Creating a Tree Revival System
This will show you how to write a Tree Revival System in JASS.
Please give some kind of credit if you use this system.
Question:
I don't know JASS can you make a GUI one?
Answer:
If you don't know JASS it's OK. I explained everything: you can't possibly make a mistake... if you do try to copy and paste. Or use the included sample map.
Copy and Paste these functions below into your map's custom header.
This may look kind of confusing but I will explain what it does.
This is VERY vital to the system. It starts it all. How does it do it you ask?
Simple: it Picks Every Destructible In The Playable Map...and then it calls the function Trig_Int_Tree Revival.
This is the rawcode for every single tree in Warcraft...you can add your own easily by pressing CTRl+D while looking at the Object Editor
//then follow the same format and add your tree's RAWCODE inside single quotes (Example ' ' )
This is pretty simple also. It is called at Map Initialization. What it does is create a local trigger IF the destructible is a tree. This is found out by using the function IsDesTree which is found in this tutorial, "t" in this case. It adds the Event a Destructable dies and adds an action. Actually it will call function RegrowTrees everytime the event is activated.
What this does is to get the dying destructable(s) and sets it to local destructable tree. It then waits TreeRegrowTime seconds..if you don't know what this is yet...it will be explained. After the wait is done it will Revive the Destructable SHOWING birth animations. If you don't want it to show birth animations then set the TRUE to FALSE.
This constant function is the Wait that is used in the RegrowTrees function.
It returns 10.00 seconds to wait. This return can never change while the system is running. If you want the trees to revive after a different alloted time then edit the 10.00 to whatever number you please.
Example:
I just editted the 10.00 to 30.00 now after 30.00 seconds the trees will respawn instead of after 10.00 seconds.
If you followed the tutorial you should have this in your map header by now.
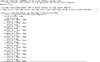
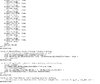
Now comes the easy part...
Inside your Map Initialization trigger add the action:
Ok..so here is a screen shot of the Map Initialization trigger in the sample map.
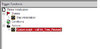
Thanks for reading this tutorial. I hope it helped you learn how to create a tree revival system in JASS.
-Hero
Sample Map: View attachment Tree Revival System.w3x
This will show you how to write a Tree Revival System in JASS.
Please give some kind of credit if you use this system.
Question:
I don't know JASS can you make a GUI one?
Answer:
If you don't know JASS it's OK. I explained everything: you can't possibly make a mistake... if you do try to copy and paste. Or use the included sample map.
Copy and Paste these functions below into your map's custom header.
JASS:
constant function TreeRegrowTime takes nothing returns real
return 10.00 //the amount of time you want before the tree respawns
endfunction
//To add more trees press CTRl+D while looking at the Object Editor
//then follow the same format and add your tree's RAWCODE inside single quotes (Example ' ' )
function IsDesTree takes destructable a returns boolean
local integer d=GetDestructableTypeId(a)
if d =='ATtr' then
return true
elseif d=='BTtw' then
return true
elseif d=='KTtw' then
return true
elseif d=='YTft' then
return true
elseif d=='JTct' then
return true
elseif d=='YTst' then
return true
elseif d=='YTct' then
return true
elseif d=='YTwt' then
return true
elseif d=='JTwt' then
return true
elseif d=='DTsh' then
return true
elseif d=='FTtw' then
return true
elseif d=='CTtr' then
return true
elseif d=='ITtw' then
return true
elseif d=='NTtw' then
return true
elseif d=='OTtw' then
return true
elseif d=='ZTtw' then
return true
elseif d=='WTst' then
return true
elseif d=='LTlt' then
return true
elseif d=='GTsh' then
return true
elseif d=='Xtlt' then
return true
elseif d=='WTtw' then
return true
elseif d=='Attc' then
return true
elseif d=='BTtc' then
return true
elseif d=='CTtc' then
return true
elseif d=='ITtc' then
return true
elseif d=='NTtc' then
return true
elseif d=='ZTtc' then
return true
else
return false
endif
endfunction
function RegrowTrees takes nothing returns nothing
local destructable tree=GetDyingDestructable()
call TriggerSleepAction(TreeRegrowTime())
call DestructableRestoreLife( tree, GetDestructableMaxLife(tree), true )
set tree=null
endfunction
function Trig_Int_Tree_Revival takes nothing returns nothing
local trigger t
if IsDesTree(GetEnumDestructable())==true then
set t=CreateTrigger()
call TriggerRegisterDeathEvent( t, GetEnumDestructable() )
call TriggerAddAction(t,function RegrowTrees)
endif
endfunction
function Int_Tree_Revive takes nothing returns nothing
call EnumDestructablesInRectAll( GetPlayableMapRect(), function Trig_Int_Tree_Revival )
endfunction
This may look kind of confusing but I will explain what it does.
JASS:
function Int_Tree_Revive takes nothing returns nothing
call EnumDestructablesInRectAll( GetPlayableMapRect(), function Trig_Int_Tree_Revival )
endfunction
This is VERY vital to the system. It starts it all. How does it do it you ask?
Simple: it Picks Every Destructible In The Playable Map...and then it calls the function Trig_Int_Tree Revival.
JASS:
function IsDesTree takes destructable a returns boolean
local integer d=GetDestructableTypeId(a)
if d =='ATtr' then
return true
elseif d=='BTtw' then
return true
elseif d=='KTtw' then
return true
elseif d=='YTft' then
return true
elseif d=='JTct' then
return true
elseif d=='YTst' then
return true
elseif d=='YTct' then
return true
elseif d=='YTwt' then
return true
elseif d=='JTwt' then
return true
elseif d=='DTsh' then
return true
elseif d=='FTtw' then
return true
elseif d=='CTtr' then
return true
elseif d=='ITtw' then
return true
elseif d=='NTtw' then
return true
elseif d=='OTtw' then
return true
elseif d=='ZTtw' then
return true
elseif d=='WTst' then
return true
elseif d=='LTlt' then
return true
elseif d=='GTsh' then
return true
elseif d=='Xtlt' then
return true
elseif d=='WTtw' then
return true
elseif d=='Attc' then
return true
elseif d=='BTtc' then
return true
elseif d=='CTtc' then
return true
elseif d=='ITtc' then
return true
elseif d=='NTtc' then
return true
elseif d=='ZTtc' then
return true
else
return false
endif
endfunction
//then follow the same format and add your tree's RAWCODE inside single quotes (Example ' ' )
JASS:
function Trig_Int_Tree_Revival takes nothing returns nothing
local trigger t
if IsDesTree(GetEnumDestructable())==true then
set t=CreateTrigger()
call TriggerRegisterDeathEvent( t, GetEnumDestructable() )
call TriggerAddAction(t,function RegrowTrees)
endif
endfunction
This is pretty simple also. It is called at Map Initialization. What it does is create a local trigger IF the destructible is a tree. This is found out by using the function IsDesTree which is found in this tutorial, "t" in this case. It adds the Event a Destructable dies and adds an action. Actually it will call function RegrowTrees everytime the event is activated.
JASS:
function RegrowTrees takes nothing returns nothing
local destructable tree=GetDyingDestructable()
call TriggerSleepAction(TreeRegrowTime())
call DestructableRestoreLife( tree, GetDestructableMaxLife(tree), true )
set tree=null
endfunction
What this does is to get the dying destructable(s) and sets it to local destructable tree. It then waits TreeRegrowTime seconds..if you don't know what this is yet...it will be explained. After the wait is done it will Revive the Destructable SHOWING birth animations. If you don't want it to show birth animations then set the TRUE to FALSE.
JASS:
constant function TreeRegrowTime takes nothing returns real
return 10.00 //the amount of time you want before the tree respawns
endfunction
This constant function is the Wait that is used in the RegrowTrees function.
It returns 10.00 seconds to wait. This return can never change while the system is running. If you want the trees to revive after a different alloted time then edit the 10.00 to whatever number you please.
Example:
JASS:
constant function TreeRegrowTime takes nothing returns real
return 30.00 //the amount of time you want before the tree respawns
endfunction
I just editted the 10.00 to 30.00 now after 30.00 seconds the trees will respawn instead of after 10.00 seconds.
If you followed the tutorial you should have this in your map header by now.
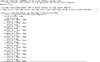
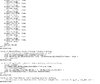
Now comes the easy part...
Inside your Map Initialization trigger add the action:
JASS:
Custom script: call Int_Tree_Revive()
Ok..so here is a screen shot of the Map Initialization trigger in the sample map.
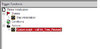
Thanks for reading this tutorial. I hope it helped you learn how to create a tree revival system in JASS.
-Hero
Sample Map: View attachment Tree Revival System.w3x
Last edited by a moderator: