- Joined
- Oct 9, 2019
- Messages
- 295
As the title says: this custom spell when it hits many targets causes unrecoverable lag which freezes the game
Systems used can be found here:
www.hiveworkshop.com
www.hiveworkshop.com
www.hiveworkshop.com
www.hiveworkshop.com
www.hiveworkshop.com
I suspect its call LightningUtils.enumUnits(g, beam, 100.) that's doing it since nothing else in my map uses that but idk why.
JASS:
scope LaserSweep
private struct Missile extends Missiles
static integer AID = 'A000'
static real angleincrement = 9.0
real angle
static real distance = 1200.
unit dummytarget
real anglecovered
static real anglecoveredmax = 45.
group alreadyhit
Lightning beam
method onFinish takes nothing returns boolean
call DummyAddRecycleTimer(dummytarget,0.5)
set dummytarget=null
if UnitAlive(source) and anglecovered < anglecoveredmax then
set angle = angle + angleincrement
set anglecovered = anglecovered + angleincrement
set .dummytarget = GetRecycledDummyAnyAngle(GetUnitX(source) + distance * Cos(angle * bj_DEGTORAD),GetUnitY(source) + distance * Sin(angle * bj_DEGTORAD),0)
call deflectTarget(dummytarget)
return false
endif
call DestroyGroup(alreadyhit)
call beam.remove()
return true
endmethod
method onPeriod takes nothing returns boolean
local group g = CreateGroup()
local unit u
call LightningUtils.enumUnits(g, beam, 100.)
loop
set u=FirstOfGroup(g)
exitwhen u==null
if not IsUnitInGroup(u,alreadyhit) and IsUnitEnemy(u, owner) and not IsUnitType(u, UNIT_TYPE_MAGIC_IMMUNE) and not BlzIsUnitInvulnerable(u) and UnitAlive(u) and not IsUnitType(u,UNIT_TYPE_STRUCTURE) then
call GroupAddUnit(alreadyhit,u)
call UnitDamageTarget(source, u, damage, false, true, ATTACK_TYPE_NORMAL, DAMAGE_TYPE_MAGIC, null)
call AddUnitBonusTimed(u,BONUS_ARMOR,-5,30.0)
endif
call GroupRemoveUnit(g,u)
endloop
set beam.targetX = .x
set beam.targetY = .y
call DestroyGroup(g)
set g = null
return false
endmethod
static method onCast takes nothing returns nothing
local unit c = GetTriggerUnit()
local real x = GetUnitX(c)
local real y = GetUnitY(c)
local real startingangle = GetUnitFacing(c) - (anglecoveredmax / 2)
local real startingx = x + distance * Cos(startingangle * bj_DEGTORAD)
local real startingy = y + distance * Sin(startingangle * bj_DEGTORAD)
local thistype this = thistype.create(startingx, startingy, 50.0, startingx,startingy, 50.0)
set .dummytarget = GetRecycledDummyAnyAngle(startingx,startingy, 0.)
set .target = dummytarget
set source = c
set owner = GetOwningPlayer(c)
set model = ""
set speed = 1000
set arc = 0.
set curve = 0.
set damage = 200 + (200 * GetUnitAbilityLevel(c,AID))
set .angle = GetUnitFacing(c)
set .anglecovered = 0.
set .beam = Lightning.unitToPoint(c,startingx,startingy,50,50.,false,0.,"DRAL",0)
set .alreadyhit = CreateGroup()
call launch()
set c=null
endmethod
static method onInit takes nothing returns nothing
call RegisterSpellEffectEvent(AID, function thistype.onCast)
endmethod
endstruct
endscope
Systems used can be found here:
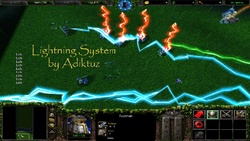
Lightning System v1.03
Basically, its a system that manages lightning creation and has useful add-ons like event handlers and the possibility of attaching any custom data to the lightnings... Features and how to use is at the code header below... :) /* Lightning System v1.03 by Adiktuz...

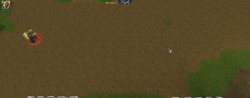
Relativistic Missiles [vJASS][LUA][GUI]
Relativistic Missiles v2.8 by Chopinski Introduction First of all, Credits and thanks to BPower, Dirac, and Vexorian for their missile systems at which I based this system upon, and to AGD for the help with effect orientation methods. This is a Missile system that I put together...

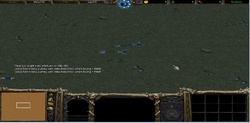
Dummy Recycler v1.25
library DummyRecycler /* // DummyRecycler v1.25 // by Flux // // A system that recycles dummy units while considering their facing angle. // It can be used as attachment dummies for visual effects or as dummy caster. // // Why is recycling a...

[Extension] Lightning Utils
Well, basically its a library containing additional tools for my Lightning System Uploaded it here as Magtheridon96 suggested ->Obtain units between the Lightning ->Set color/alpha gradient for Timed Lightnings /* Lightning Utilities v1.01 by Adiktuz A set of methods...

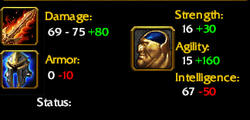
New Bonus [vJASS][LUA]
Intro Hello, this is my first submission in the spell section, so apologies in advance for any mistake. I present to you the New Bonus System. Since Object Merger stopped working and we still have no ways to edit the bonus values of a unit natively, I decided to create this light weight system...

I suspect its call LightningUtils.enumUnits(g, beam, 100.) that's doing it since nothing else in my map uses that but idk why.
Last edited: