- Joined
- Mar 6, 2006
- Messages
- 9,240
I am making a spell request that has a moving laser beam. I need to check to know when it collides with units. So I check whether the unit is inside a rectangle like this:
Here's the code:
How to make the library better? Anything wrong with it?
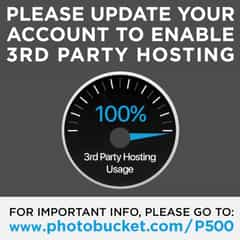
Here's the code:
JASS:
library PointInRect
// Returns a boolean that tells whether the point is inside a rectangle.
// (px , py) = Point to check
// (cx , cy) = lower left corner of the rect
// (ax , ay) = upper left corner
// (bx , by) = lower right corner
function IsPointInRect takes real px , real py , real cx , real cy , real ax , real ay , real bx , real by returns boolean
// Vector from lower left corner to point
local real v_angle = Atan2( py - cy , px - cx )
local real v_length = SquareRoot( (py-cy)*(py-cy) + (px-cx)*(px-cx) )
// Vector from lower left corner to upper left corner
local real v1_angle = Atan2( ay - cy , ax - cx )
local real v1_length = SquareRoot( (ay-cy)*(ay-cy) + (ax-cx)*(ax-cx) )
// Vector from lower left corner to lower right corner
local real v2_angle = Atan2( by - cy , bx - cx )
local real v2_length = SquareRoot( (by-cy)*(by-cy) + (bx-cx)*(bx-cx) )
local real dot1 = v_length*v1_length*Cos(v_angle-v1_angle)
local real dot2 = v1_length*v1_length
local real dot3 = v_length*v2_length*Cos(v_angle*v2_angle)
local real dot4 = v2_length*v2_length
return dot1 >= 0 and dot1 <= dot2 and dot3 >= 0 and dot3 <= dot4
endfunction
endlibrary
JASS:
library PointInRect
// Returns a boolean that tells whether the point is inside a rectangle.
// (px , py) = Point to check
// (cx , cy) = lower left corner of the rect
// (ax , ay) = upper left corner
// (bx , by) = lower right corner
function IsPointInRect takes real px , real py , real cx , real cy , real ax , real ay , real bx , real by returns boolean
local real dot1 = (px-cx)*(ax-cx) + (py-cy)*(ay-cy)
local real dot2 = (ax-cx)*(ax-cx) + (ay-cy)*(ay-cy)
local real dot3 = (px-cx)*(bx-cx) + (py-cy)*(by-cy)
local real dot4 = (bx-cx)*(bx-cx) + (by-cy)*(by-cy)
return dot1 >= 0 and dot1 <= dot2 and dot3 >= 0 and dot3 <= dot4
endfunction
endlibrary
How to make the library better? Anything wrong with it?
Last edited: