- Joined
- Feb 12, 2012
- Messages
- 413
Table of contents
•What you need
•Ready
•Start
-----------------
•Get Angle
•Get Distance
•Get smallest distance and count
Sometimes, you're doing something, and to complete it, you need to create an arrow (Just example) but you don't know how to create it and you are lazy to create one by one..
I have a solution to help you:
So...
How to turn this:
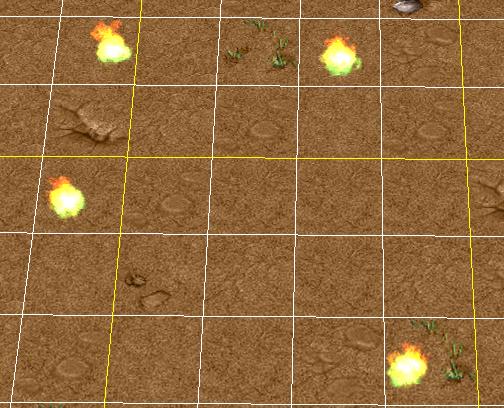
Into this:
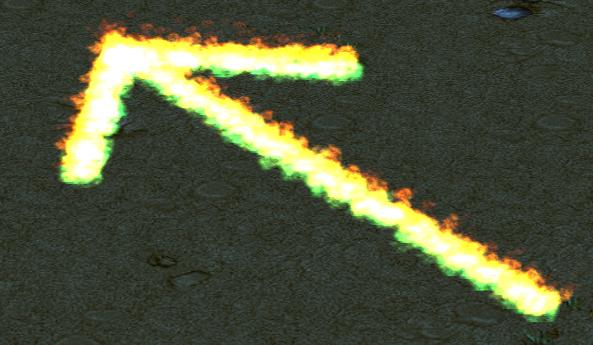
I/ What you need
So, you need to generate points and turn it into the shape you want
Like this:
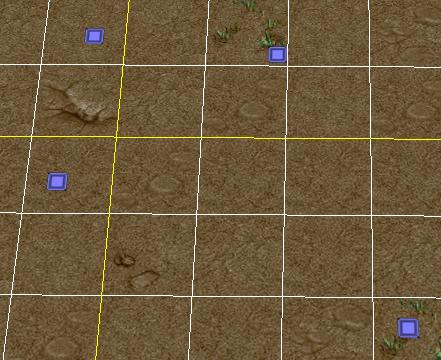
You can use anything you want not necessarily the rect. Just know that the point xy.
II/ Ready
Well, you must remember two thing: Angle and Distance, i don't want to tell you what is that..
NOW EXAMPLE:
Suppose i have 4 rect:
• Rect 1: gg_rct_1 (left point)
• Rect 2: gg_rct_2 (up point)
• Rect 3: gg_rct_3 (right point)
• Rect 4: gg_rct_4 (down point)
A/ Get angle
Well, You need to know the angle between the two points for each, but how ?, here's your answer:
-JASS-
local real a = Atan2(GetRectCenterY(gg_rct_4) - GetRectCenterY(gg_rct_2), GetRectCenterX(gg_rct_4) - GetRectCenterX(gg_rct_2))
-GUI-
-
Set LEAKS = (Center of 2 <gen>)
-
Set LEAKS1 = (Center of 4 <gen>)
-
Set Angle = (Angle from LEAKS to LEAKS1)
You're done !
B/ Get distance
You must get the distance between two point too, like this:
-JASS-
local real dx = GetRectCenterX(gg_rct_4) - GetRectCenterX(gg_rct_2)
local real dy = GetRectCenterY(gg_rct_4) - GetRectCenterY(gg_rct_2)
local real distance = SquareRoot(dx * dx + dy * dy)
-GUI-
-
Set Distance = (Distance between LEAKS and LEAKS1)
IMPORTANT STAGE: You must have count of <effect,unit or stuff> you using to make this arrow, for example, i want to create 20 effect, like this:
-JASS-
local integer i = 20
-GUI-
-
Set Count = 20
-JASS-
local real logic = distance/i
-GUI-
-
Set MinDistance = (Distance / (Real(Count)))
C/ START
Now you must write the loop, like this:
-JASS-
JASS:
local real d = 0.
local real cos = Cos(a)
local real sin = Sin(a)
loop
exitwhen i == 0
set x = GetRectCenterX(gg_rct_2) + d * cos
set y = GetRectCenterY(gg_rct_2) + d * sin
call AddSpecialEffect("Abilities\\Spells\\Human\\FlameStrike\\FlameStrikeDamageTarget.mdl",x,y)
set d = d + logic
set i = i - 1
endloop
-GUI-
-
For each (Integer A) from 1 to Count, do (Actions)
-
Loop - Actions
-
Set DistanceInc = (DistanceInc + MinDistance)
-
Special Effect - Create a special effect at (LEAKS offset by DistanceInc towards Angle degrees) using Abilities\Spells\Human\FlameStrike\FlameStrikeDamageTarget.mdl
-
-
-
Custom script: call RemoveLocation(udg_LEAKS1)
"Abilities\\Spells\\Human\\FlameStrike\\FlameStrikeDamageTarget.mdl" is string model of effect i want to use, d is value increases every i subtract, a is Angle you did the previous.
Here is the results
-JASS-
JASS:
local integer i = 20
local real x
local real y
local real dx = GetRectCenterX(gg_rct_4) - GetRectCenterX(gg_rct_2)
local real dy = GetRectCenterY(gg_rct_4) - GetRectCenterY(gg_rct_2)
local real distance = SquareRoot(dx * dx + dy * dy)
local real d = 0.
local real logic = distance/i
local real a = Atan2(GetRectCenterY(gg_rct_4) - GetRectCenterY(gg_rct_2), GetRectCenterX(gg_rct_4) - GetRectCenterX(gg_rct_2))
local real cos = Cos(a)
local real sin = Sin(a)
//First arrow
loop
exitwhen i == 0
set x = GetRectCenterX(gg_rct_2) + d * cos
set y = GetRectCenterY(gg_rct_2) + d * sin
call AddSpecialEffect("Abilities\\Spells\\Human\\FlameStrike\\FlameStrikeDamageTarget.mdl",x,y)
set d = d + logic
set i = i - 1
endloop
-
Actions
-
Set DistanceInc = 0.00
-
Set LEAKS = (Center of 2 <gen>)
-
Set LEAKS1 = (Center of 4 <gen>)
-
Set Angle = (Angle from LEAKS to LEAKS1)
-
Set Distance = (Distance between LEAKS and LEAKS1)
-
Set Count = 20
-
Set MinDistance = (Distance / (Real(Count)))
-
Custom script: call RemoveLocation(udg_LEAKS1)
-
For each (Integer A) from 1 to Count, do (Actions)
-
Loop - Actions
-
Set DistanceInc = (DistanceInc + MinDistance)
-
Set LEAKS1 = (LEAKS offset by DistanceInc towards Angle degrees)
-
Special Effect - Create a special effect at LEAKS1 using Abilities\Spells\Human\FlameStrike\FlameStrikeDamageTarget.mdl
-
Custom script: call RemoveLocation(udg_LEAKS1)
-
-
-
Custom script: call RemoveLocation(udg_LEAKS)
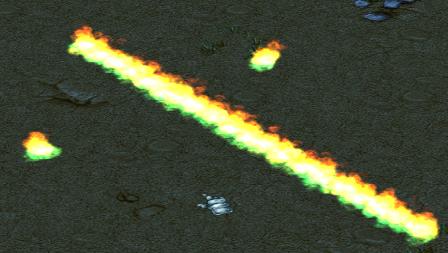
And you do the same with the gg_rct_1 and gg_rct_3
And you will get the arrow like me ^^!
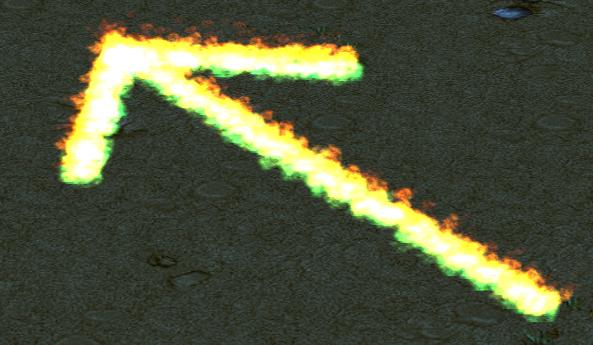
So if the angle and distance change in game, what can i do ??.....
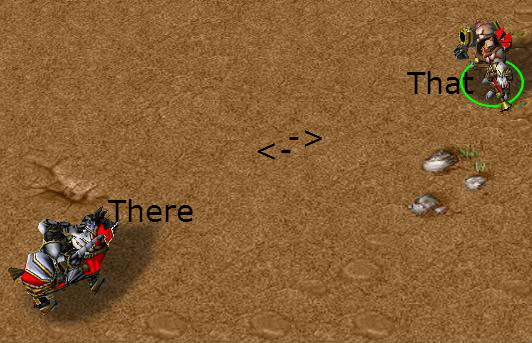
You must do the same thing like that
-JASS-
JASS:
function Trig_Untitled_Trigger_001_Actions takes nothing returns nothing
local real x = GetUnitX(GetTriggerUnit())
local real y = GetUnitY(GetTriggerUnit())
local real o = GetUnitX(GetSpellTargetUnit())
local real z = GetUnitY(GetSpellTargetUnit())
local real dx = x-o
local real dy = y-z
local real distance = SquareRoot(dx * dx + dy * dy)
local real angle = Atan2(z - y, o - x)
local integer i = 20
local real min = distance/i
local real m = 0.
local real cos = Cos(angle)
local real sin = Sin(angle)
loop
exitwhen i < 0
set m = m + min
set o = x+m*cos
set z = y+m*sin
call AddSpecialEffect("Abilities\\Spells\\Other\\BreathOfFire\\BreathOfFireDamage.mdl",o,z)
set i = i - 1
endloop
endfunction
function a takes nothing returns boolean
return GetSpellAbilityId() == 'A000'
endfunction
//===========================================================================
function InitTrig_JASS takes nothing returns nothing
set gg_trg_JASS = CreateTrigger( )
call TriggerRegisterAnyUnitEventBJ( gg_trg_JASS, EVENT_PLAYER_UNIT_SPELL_EFFECT )
call TriggerAddCondition(gg_trg_JASS,Condition(function a))
call TriggerAddAction( gg_trg_JASS, function Trig_Untitled_Trigger_001_Actions )
endfunction
-GUI-
-
Events
-
Unit - A unit Starts the effect of an ability
-
Conditions
-
(Ability being cast) Equal to Special Effect - GUI [W]
-
-
Actions
-
Set Point = (Position of (Triggering unit))
-
Set Point1 = (Position of (Target unit of ability being cast))
-
Set Angle = (Angle from Point to Point1)
-
Set Distance = (Distance between Point and Point1)
-
Set Count = 20
-
Set Min = (Distance / (Real(Count)))
-
Set M = 0.00
-
Custom script: call RemoveLocation(udg_Point1)
-
For each (Integer A) from 1 to Count, do (Actions)
-
Loop - Actions
-
Set M = (M + Min)
-
Set Point1 = (Point offset by M towards Angle degrees)
-
Special Effect - Create a special effect at Point1 using Abilities\Spells\Other\BreathOfFire\BreathOfFireDamage.mdl
-
Custom script: call RemoveLocation(udg_Point1)
-
-
-
Custom script: call RemoveLocation(udg_Point)
-
-
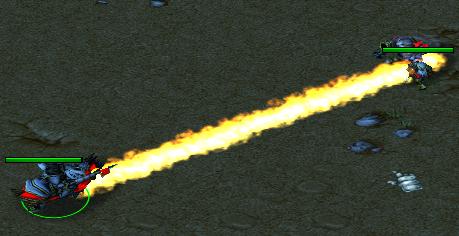
Attachments
-
untit226.jpg48.2 KB · Views: 201
-
untit227.jpg31.9 KB · Views: 182
-
untit228.jpg34 KB · Views: 210
-
untit229.jpg17.9 KB · Views: 170
-
untitl32.jpg46.6 KB · Views: 177
-
untitl34.jpg18.1 KB · Views: 201
-
Arrow GUI.w3x17.6 KB · Views: 146
-
Arrow.w3x17.2 KB · Views: 132
-
Dis and Ang GUI and JASS.w3x18.3 KB · Views: 128
Last edited by a moderator: