- Joined
- Oct 20, 2007
- Messages
- 353
This system allows you creating, showing/hiding, deleting multiboard groups.
You can switch multiboards ("tabs") from group by clicking "minimize" button.
On the following screenshot you can see group with 3 multiboards in all possible four states (each multiboard and minimized state):
Multiboard Group library:
Testing trigger:
This trigger creates multiboard group from screenshot.
Test map: View attachment MultiboardGroup.w3x
You can switch multiboards ("tabs") from group by clicking "minimize" button.
On the following screenshot you can see group with 3 multiboards in all possible four states (each multiboard and minimized state):
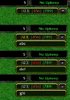
Multiboard Group library:
JASS:
library MultiboardGroupLibrary initializer Init // by D.O.G. version 2.1
// Settings
globals
// Default color of selected tab's name (active multiboard's name)
private string DEFAULT_ACTIVE_COLOR = "|cFFFFCC00"
// Default color of unselected tabs' names
private string DEFAULT_INACTIVE_COLOR = "|cFFC0C0C0"
private string SPACING_COLOR = "|cFF707070" // Color of text before, between and after tabs' names
private string SPACING_LEFT = "[" // Text before tabs' names
private string SPACING_CENTER = "] [" // Text between tabs' names
private string SPACING_RIGHT = "]" // Text after tabs' names
private constant real CHECK_PERIOD = 0.01 // Time interval in which multiboard state is checked
endglobals
// Do not edit
globals
private integer ActiveGroup = -1
private integer LastGroup = -1
private hashtable Hash = InitHashtable()
endglobals
////////////////////////
function GetMultiboardCountInGroup takes integer mgroup returns integer
return LoadInteger(Hash, mgroup, -2)
endfunction
private function SetMultiboardCountInGroup takes integer mgroup, integer count returns nothing
call SaveInteger(Hash, mgroup, -2, count)
endfunction
////////////////////////
function GetMultiboardNameInGroup takes integer mgroup, integer index returns string
return LoadStr(Hash, mgroup, index)
endfunction
function SetMultiboardNameInGroup takes integer mgroup, integer index, string name returns nothing
call SaveStr(Hash, mgroup, index, name)
endfunction
///////////////////////////
function GetActiveMultiboardInGroup takes integer mgroup returns integer
return LoadInteger(Hash, mgroup, -3)
endfunction
function SetActiveMultiboardInGroup takes integer mgroup, integer index returns nothing
call SaveInteger(Hash, mgroup, -3, index)
endfunction
///////////////////////
function GetMultiboardNameColorInGroup takes integer mgroup, integer index returns string
return LoadStr(Hash, mgroup, index + 256)
endfunction
function SetMultiboardNameColorInGroup takes integer mgroup, integer index, string color returns nothing
call SaveStr(Hash, mgroup, index + 256, color)
endfunction
///////////////////////
function GetMultiboardNameInactiveColorInGroup takes integer mgroup, integer index returns string
return LoadStr(Hash, mgroup, index + 512)
endfunction
function SetMultiboardNameInactiveColorInGroup takes integer mgroup, integer index, string color returns nothing
call SaveStr(Hash, mgroup, index + 512, color)
endfunction
///////////////////////
function GetMultiboardFromGroup takes integer mgroup, integer mindex returns multiboard
return LoadMultiboardHandle(Hash, mgroup, mindex)
endfunction
private function SetMultiboardToGroup takes integer mgroup, integer mindex, multiboard mb returns nothing
call SaveMultiboardHandle(Hash, mgroup, mindex, mb)
endfunction
///////////////////////
function AddMultiboardToGroupC takes integer mgroup, multiboard mb, string activecolor, string inactivecolor returns nothing
//local multiboard mb0
local integer mcount = GetMultiboardCountInGroup(mgroup)
/*if mcount == 0 then
set mb0 = CreateMultiboard()
call SetMultiboardToGroup(mgroup, 0, mb0)
set mcount = 1
set mb0 = null
endif*/
call SetMultiboardToGroup(mgroup, mcount, mb)
call SetMultiboardNameInGroup(mgroup, mcount, MultiboardGetTitleText(mb))
call SetMultiboardNameColorInGroup(mgroup, mcount, activecolor)
call SetMultiboardNameInactiveColorInGroup(mgroup, mcount, inactivecolor)
call SetMultiboardCountInGroup(mgroup, mcount + 1)
endfunction
function AddMultiboardToGroup takes integer mgroup, multiboard mb returns nothing
call AddMultiboardToGroupC(mgroup, mb, DEFAULT_ACTIVE_COLOR, DEFAULT_INACTIVE_COLOR)
endfunction
function NewMultiboardGroup takes nothing returns integer
set LastGroup = LastGroup + 1
call SetMultiboardToGroup(LastGroup, 0, CreateMultiboard())
call SetMultiboardCountInGroup(LastGroup, 1)
return LastGroup
endfunction
function UpdateMultiboardNamesInGroup takes integer mgroup returns nothing
local integer mlast = GetMultiboardCountInGroup(mgroup) - 1
local string array n
local string array t
local integer i = 1
local integer j
set n[0] = SPACING_COLOR + SPACING_LEFT + "|r"
loop
exitwhen i >= mlast
set n[i] = GetMultiboardNameInGroup(mgroup, i) + "|r" + SPACING_COLOR + SPACING_CENTER + "|r"
set i = i + 1
endloop
set n[mlast] = GetMultiboardNameInGroup(mgroup, i) + "|r" + SPACING_COLOR + SPACING_RIGHT + "|r"
set i = 0
loop
exitwhen i > mlast
set j = 0
loop
exitwhen j > mlast
if i == j then
set t[i] = t[i] + GetMultiboardNameColorInGroup(mgroup, j) + n[j]
else
set t[i] = t[i] + GetMultiboardNameInactiveColorInGroup(mgroup, j) + n[j]
endif
set j = j + 1
endloop
set i = i + 1
endloop
set i = 0
loop
exitwhen i > mlast
call MultiboardSetTitleText(GetMultiboardFromGroup(mgroup, i), t[i])
set i = i + 1
endloop
endfunction
function ShowMultiboardGroup takes integer mgroup returns nothing
local integer i = GetActiveMultiboardInGroup(mgroup)
local multiboard t = GetMultiboardFromGroup(mgroup, i)
set ActiveGroup = mgroup
call MultiboardDisplay(t, true)
call MultiboardMinimize(t, i == 0)
set t = null
endfunction
function ShowMultiboardGroupToPlayer takes integer mgroup, player p returns nothing
if p == GetLocalPlayer() then
call ShowMultiboardGroup(mgroup)
endif
endfunction
function HideCurrentMultiboardGroup takes nothing returns nothing
call MultiboardDisplay(GetMultiboardFromGroup(ActiveGroup, GetActiveMultiboardInGroup(ActiveGroup)), false)
set ActiveGroup = -1
endfunction
function HideCurrentMultiboardGroupForPlayer takes player p returns nothing
if p == GetLocalPlayer() then
call HideCurrentMultiboardGroup()
endif
endfunction
function RemoveMultiboardGroup takes integer mgroup returns nothing
if mgroup == ActiveGroup then
call HideCurrentMultiboardGroup()
endif
call FlushChildHashtable(Hash, mgroup)
endfunction
private function Mod takes integer a, integer b returns integer
if (a >= 0) and (a < b) then
return a
endif
return 0
endfunction
private function Handler takes nothing returns nothing
local integer act
local multiboard mb
if ActiveGroup != -1 then
set act = GetActiveMultiboardInGroup(ActiveGroup)
if (act != 0) == IsMultiboardMinimized(GetMultiboardFromGroup(ActiveGroup, act)) then
set act = Mod(act + 1, GetMultiboardCountInGroup(ActiveGroup))
call SetActiveMultiboardInGroup(ActiveGroup, act)
set mb = GetMultiboardFromGroup(ActiveGroup, act)
call MultiboardDisplay(mb, true)
call MultiboardMinimize(mb, act == 0)
endif
endif
set mb = null
endfunction
private function Init takes nothing returns nothing
call TimerStart(CreateTimer(), CHECK_PERIOD, true, function Handler)
endfunction
endlibrary
Testing trigger:
This trigger creates multiboard group from screenshot.
JASS:
library MultiboardGroupTest initializer Init requires MultiboardGroupLibrary
private function CreateMultiboard1x1 takes string title, string text returns multiboard
local multiboard mb = CreateMultiboard()
local multiboarditem mbi
call MultiboardSetRowCount(mb, 1)
call MultiboardSetColumnCount(mb, 1)
call MultiboardSetTitleText(mb, title)
set mbi = MultiboardGetItem(mb, 0, 0)
call MultiboardSetItemValue(mbi, text)
call MultiboardSetItemStyle(mbi, true, false)
call MultiboardReleaseItem(mbi)
set mbi = null
return mb
endfunction
private function Actions takes nothing returns nothing
local integer g = NewMultiboardGroup()
call AddMultiboardToGroup(g, CreateMultiboard1x1("123", "abc"))
call AddMultiboardToGroupC(g, CreateMultiboard1x1("456", "def"), "|cFFFF0000", "|cFFBB4444")
call AddMultiboardToGroupC(g, CreateMultiboard1x1("789", "ghi"), "|cFF00FF00", "|cFF44BB44")
call UpdateMultiboardNamesInGroup(g)
call ShowMultiboardGroup(g)
call DestroyTimer(GetExpiredTimer())
endfunction
private function Init takes nothing returns nothing
call TimerStart(CreateTimer(), 0.1, false, function Actions)
endfunction
endlibrary
Test map: View attachment MultiboardGroup.w3x
Attachments
Last edited: