- Joined
- Aug 3, 2005
- Messages
- 745
Nether Swap
Instantly swaps location with target unit:
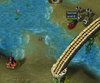
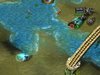
Intro
This is a very basic and simplistic spell, which makes it ideal for starting out with jass.
This tutorial does not cover the introduction to jass but rather goes straight into making a spell.
It also has a brief introduction to the Channel ability
Starters
Firstly go into the object editor and create a nether swap ability.
I recommend basing it of Channel, which is found under Neutral Hostile> Hero Abilities
Here is a screenshot to show how you should modify it
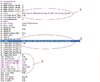
1 & 2 - These are the special effects that will be added to Caster and Target, I chose Blink, its up to you.
3 - Make sure you have the same options.
> Disable other abilities = false
> Follow Through Time = 0.00
> Options = Visible
> Target Type = Unit
Next we need to create the trigger
Go into the Trigger Editor and create a trigger called: Nether Swap
Please Note: NOT NetherSwap or nether swap etc.but Nether Swap
Should look like this:
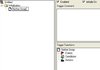
Next you need to converted it to custom script:
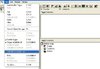
For now we will skip the Conditions/Actions bit as this is quite boring, Once weve made a few spells I'll explain this to you
So Ill give you a base code to copy paste.
This is what you should currently have:

Copy Paste this:
JASS:
function Trig_Nether_Swap_Conditions takes nothing returns boolean
return GetSpellAbilityId() == 'A00P'
endfunction
function Trig_Nether_Swap_Actions takes nothing returns nothing
endfunction
//===========================================================================
function InitTrig_Nether_Swap takes nothing returns nothing
set gg_trg_Nether_Swap = CreateTrigger()
call TriggerRegisterAnyUnitEventBJ(gg_trg_Nether_Swap, EVENT_PLAYER_UNIT_SPELL_EFFECT)
call TriggerAddCondition(gg_trg_Nether_Swap, Condition(function Trig_Nether_Swap_Conditions))
call TriggerAddAction(gg_trg_Nether_Swap, function Trig_Nether_Swap_Actions)
endfunction
So now it should look like this:
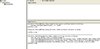
Now we need to make sure the correct ability is in the condition.
For this go back into the Object Editor and click
View > Display Values as Raw Data
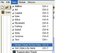
Next we need to get the raw data for the ability
In this case it is A000
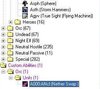
Note: In your map if may differ so make sure to check.
Next go back into the trigger editor and lets alter the condition

Notice how Ive changed it to 'A000'
Ok we are now ready to start coding the spell
Method
This is how we will do the spell:
- Create a local to store: Casting Unit
- Create a local to store: Target Unit of Ability Being Cast Unit
- Create locals to store: Casting Unit's co-ordinates
- Create locals to store: Target Unit of Ability Being Cast's co-ordinates
- Move Casting Unit
- Move Target Unit of ability being cast
- Clean up leaks
Jass Code
The following code will go inside the Actions section:

Where the cursor is begin typing in there
Firstly we declare the locals
JASS:
local unit c = GetTriggerUnit()
local unit t = GetSpellTargetUnit()
c = the Casting Unit
t = The Target Unit of ability being cast
Next we create locals for their co-ordinates
JASS:
local real xc = GetUnitX(c)
local real yc = GetUnitY(c)
local real xt = GetUnitX(t)
local real yt = GetUnitY(t)
xc & yc = the co-ordinates of the Casting Unit
xt & yt = the co-ordinates of the Target Unit of ability being cast
Now we will set each unit's new position
JASS:
call SetUnitX(c, xt)
call SetUnitY(c, yt)
call SetUnitX(t, xc)
call SetUnitY(t, yc)
This will place:
- Casting unit at co-ordinates stored for the Target Unit of ability being cast
- Target Unit of ability being cast at co-ordinates stored for the Casting Unit
Finally we clean up the leaks
JASS:
set c = null
set t = null
This is overall how your code should look
JASS:
function Trig_Nether_Swap_Conditions takes nothing returns boolean
return GetSpellAbilityId() == 'A00P'
endfunction
function Trig_Nether_Swap_Actions takes nothing returns nothing
local unit c = GetTriggerUnit()
local unit t = GetSpellTargetUnit()
local real xc = GetUnitX(c)
local real yc = GetUnitY(c)
local real xt = GetUnitX(t)
local real yt = GetUnitY(t)
call SetUnitX(c, xt)
call SetUnitY(c, yt)
call SetUnitX(t, xc)
call SetUnitY(t, yc)
set c = null
set t = null
endfunction
//===========================================================================
function InitTrig_Nether_Swap takes nothing returns nothing
set gg_trg_Nether_Swap = CreateTrigger()
call TriggerRegisterAnyUnitEventBJ(gg_trg_Nether_Swap, EVENT_PLAYER_UNIT_SPELL_EFFECT)
call TriggerAddCondition(gg_trg_Nether_Swap, Condition(function Trig_Nether_Swap_Conditions))
call TriggerAddAction(gg_trg_Nether_Swap, function Trig_Nether_Swap_Actions)
endfunction
You can make the code, slightly more efficient by replacing:
JASS:
local real xt = GetUnitX(t)
local real yt = GetUnitY(t)
call SetUnitX(c, xt)
call SetUnitY(c, yt)
with:
JASS:
call SetUnitX(c, GetUnitX(t))
call SetUnitY(c, GetUnitY(t))
Your jass spell should now work, have fun and enjoy and stay tuned for more step by step mini jass tuts
thx
Fulla
Last edited by a moderator: