- Joined
- Dec 12, 2010
- Messages
- 2,074
I've provided a .j file with basic functions and tons of API, most of which went undocumented, so people started to ask questions. I'll try to comment every function I put there to somehow clear things out.
Common questions
Q: This function doesn't work with address, should I put handle instead?
A: Nope, forget what handle is when you're working with memory. Handle is nothing but jass-only thing.
Q: Where is X function?
A: Impossible or not required by me, therefore never been implemented. Mem hack allows pretty much everything, yet you have to know what you want and how to find it in the memory. Thats why you need CE or wait for somebody to actually dig things up. There's definitely ways to read any data, yet you maybe won't be able to set it.
Q: Will there be updates to API?
A: Sure, but can't make a date()
Q: Why there's randomly "divided by 4" and some of others arent?
A: When we started it we were using Memory[] array, which is 4-bytes based. Saying, whenever you access address 0x1000000, you need to ask Memory[0x1000000/4]. Then, after few months, we moved to full addresses, yet legacy..
Check twice if function you used takes one or another. Anyway, lets go
Constant-related API
WC3 has many constants which are known for limiting game possibilities. Most known of them is movespeed limit and attack speed limit. So first of all we tried to get rid of them.
WARNING
Some constants, which weren't declared in map's constants, like attack speed (AS) limit, are stored in READ ONLY memory section. Thanks to that, they are only loaded at game start and supposed to be constant all along. Altering them will cause your clients to have different limits than other. That's not a problem if they're gonna play your map only. But in case if player decides to join another game later he'll be disconnected as soon as he overcome default AS limit. Memory won't be repaired at map start, so he will have different limits, and that will fuck him up.
You as well can't detect whenever player leaves to run some code on his PC - he is out of reach. So, there's only 3 options for non-retarded coders:
Issue is, address we found first time seems to be irrelevant, so this functions basically useless if you want to pass 522 limit. Although you may check if it allows to change "constant" max speed value to lower numbers, dinamycally adjusting it for your needs instead of setting it up once at map start.
Same, just to change game constant
Read-only constant. Attack speed is measured in float and may be inbetween 0.2 and 5.0 by default (min & max), 1. = no changes (base attack time used). It's also known as -80% AS and 400% AS limits. You can change them to w/e you want, I can't see backstabs.
Read-only constant. Same as attack speed, attack time had it's limits. I never needed to change them, so untested.
Read-only constant. Default OP limit (operations limit) is 30k. Means after 30k of any kind of calls or increments game will kill the thread no matter if its finished or not. This function set limit to 800-900k or higher, literally unreachable no matter what you do. This will cause forgotted endless loops to stop whole WC3 yet allows you to keep functions inlined instead of re-calling it later.
Basic functions
ConvertHandle turns any given handle into the read address of object related.
ConvertPointer should be used onto things which aren't real handles - texttag, lightnings, ubserplats, etc. They have different scope in the memory, so different appoach needed. Im not sure if this func ever work tho.
Just a simple checker for a flag stored inside normal integer. Imaging it as bit-wise AND.
Are used to craft some address of 2 integer values. WC3 stores links onto objects using 2 keys, and that's only way for you to convert them to real address - using those functions.
For string handling
Speaks for themselves, yet won't be really needed for you
Unit's API
You can find more info about units in Unit's structure
This is referred to Unit+0x5C offset which contains some flags of unit, constants held some of known values.
And this is for Unit+0x20
Simple setter-getters, documented with numbers. Setting unit's armor always affects only white armor, although it's always returns white+green.
Obviously allows you to spoof unit with different ID, or get the ID value directly from memory instead of native
Turn's unit to phased stated (used by pathfinding algo for items on the ground), also known as wind walk-ing
Allows to change unit's coloring, including illusions. Yet illusions tends to get colored back to blue over time.
Take those for granted:
Unit movement's API:
Manupulate directly with Amov ability, disabling it
Use hardcoded counter of "affected by entangle/ensnare", keeping all other hardcoded side effects
Manipulate unit's bonus MS measured as % of base speed
Manipulate unit's current movespeed. Everytime unit is moving / receiving order and moving MS being re-calculated. It's measured in normalMS/32, means 522 ms == 16.3125 ms/32. You can ignore any speed limits setting value directly with that, but the issue is, as I said, it being recalculated on every order, slow, boost, etc.
Do note that units with MS over 522 tends to fall into the same issues as triggered movement - missing checkpoints in pathfinding.
Set up this unit's base movespeed independantly from unit's params stated in the editor.
Various unit API (lol im tired sorting)
Completely removes unit from being controllable by any player
Game won't redraw model until forced to, and we don't know how to force it. So far best approach is to change model before you creating unit, then he will have a new model.
Getting base abilities addresses
Enable/disable unit's detectability
Set unit's stun counter to 0, unsafe
Self-speaking
takes unit's address and returns ability address by ID. Alias for GetUnitAbility
Just what it says
Puts abilities list into hashtable
Tons of set-get for attacks
Just what it says
Map grid is a typical 2dimensional grid. Very bottom left grid is x=0 and y=0, and it inc to the right and to the top. This func set unit's coordinate to the cell. If used inproperly instantly crashes the game. This kind of movement DOESN'T counted as movement at all. For instance game won't re-draw fog around unit placed to the new area. So it's only for cases when unit shouldn't make his appearance for triggers or stuff anyway.
Self-speaking
Ez to reset/alter Timed Life duration
Unit has dozen fields with angles, not every of them are known. IDK, check yourself
Maybe "unit rendered time", idk, may be async between clients, unreliable
Various stuff with unit attack state
Various barely-useful functions
Self-explained. All of those have analogue in abilities - storm bolt, silence, doom. Yet you have to manually remove it, since no buff to do this work for you here.
Counts any kind of attack disabler
Windwalk-based abilities uses "Orb of slow" disable so user unable to turn it again. This fix set -100 to the counter ensuring ability will be always available
Explained in Unit's structure
Returns player's ID who REALLY issued order to the unit (GetTriggerPlayer() always returns owner of unit)
Ability's API
Be aware of caching: ability being only preloaded whenever it's added to any unit. Until then some functions may return 0 if ability wasn't found in game cache. Do no try to change abilities which arent' entered map yet, it have no purpose anyway.
Note: type ability is just an object made of real ability as an address. Some old functions uses "ability" type, and you may upfresh them with some little tricks, like changing params to "unit u, integer id" and finding out address to use by yourself.
Note #2: if unit has more than 1 instance of ability (lets say thanks to item) you'll only get address of last one added. To go further you'll need to adapt system to uself of refuse this manipulations at all. On the other hand, "ability" is always refer to specific ability and not last one. Guess you'll find the way.
This one is for those who are familiar with SLK-tables. X stands for the field of ability you wanna real. Like x19 = cooldown on level 1, x20 = manacost on level 1, etc etc. Note that it requires ability to be passed which is only possible inside the events like UNIT_SPELL_*
Every spell owned by any unit has it's own cast point and backswing, counted as normal castpoint of unit + cast time of skill for foreswing, and backswing of unit for backswing of spell. You can get/set any of them without affecting any other ability.
Supposed to affect ability's cast time, must be alias for Castpoint (unsure, unused)
Any ability has a cooldown object and base cooldown field. Base cooldown used to keep trace of how many seconds this ability rechargin and ONLY used to chose proper cooldown animation frame. Adding ability cooldown effectively change timing of it's availability.
Takes address of ability divided by 4 and add some seconds to cooldown left
Returns seconds of cooldown left
Takes address of ability divided by 4 and returns cooldown left
Returns timestamp of when ability will be available again. It's based on ingame timer so its not "cooldown left"
Following APIs takes only ID/address of abilities, not the type "ability"
Self-speaking, all of them takes ID as "abil"
Aliases for aforementioned funcs
Takes Abil ID
Self-speaking, takes address of ability divided by 4
Disabled: not safe unless used with PauseUnit. Button will be blacked, but current casts of that ability won’t be interrupted.
Hidden: similar to SetPlayerAbilityAvailable (false) but for a single ability, hides icon and blocks order
Disabled2: this one is used by Orb of Slow. Button is blacked, but cooldown is stil displayed.
All of those fields are counters and may keep negative/positive values as 4 bytes integer.
ShowAbilityById_Main: adds d to the "hidden"'s counter.
Used to get ability's order ID. Disregard that Im trying to reach base ability, its unneeded in fact and was my blindness. You may improve those code freely.
takes Address and CD. May be improved to takes unit, ID and CD instead. Won't work on abilities which have no order to be used - instant crash.
Self-speaking
Used to instantly cast a spell onto target/coordinates. Non-target abils takes x/y coords instead.
This funs SKIPS all kind of checks, spell blocks, mana/target filters, anything. You may cast anything on anything.
Detailed explanation may take awhile.
Personal Silence for an ability (address required)
Ability UI's API
Base functions required by following funcs
Takes ability's ID and a key. Key should be passed as integer, meaning a number from ASCII table or using '' (SetAbilityHotkeyId('A000','C'))
Tons of setters/getters for any use
Other data are also available, yet weren't needed for me.
Effects API
Be aware of, effects, as unit's models, aren't re-drawn every time, so you have to modify their color/size/facing once, right after you created it.
Trackables API
Obvious
Misc API
simple system to display text on unusual places
Antihack API
Used to detect/prevent hackers
Does what it says, instantly changes item's ID. May have side effects but won't crash you
WinAPI API
Various batching with WinAPI, allowing to open http addresses, run from CMD, writing/reading files, exporting files from MPQ, etc
sprint_f analogue from C++
ASM API
Reads registers. Barely useful unless you have memory viewer nearby,.
If placed as condition in the very first game trigger which registers every UNIT_DAMAGED event, will return proper AT, DT and Damage before reductions.
Minimap-Ping API
[/code]
Async
Camera constants API
Read only constant. Param i stands for cam index on the mouse wheel. 0 = normal camera, 1 = moved closer, and so on. Every cam has it's own distance. Numbers go from 0 to 5 for 26 and from 0 to -5 for 27. Fuck 27.
Workers
For non-experts obviously most useful is IsReplay detection which can be extracted from TestIsReplay
Sending custom packets, you won't make it run yet, believe me
Various stuff I don't use, so it's unconfirmed about stability
Data Karaulov found about missiles, can't use any of those still.
Rectangle editor by Karaulov. Amazing thing is he barely had idea about how to JASS when he created this
Every order ingame goes through one of those wrappers, so disabling them effectively blocks local player's ability to order anything.
Unsafe, reading offsets from registers to get a real blink destination
Used to find Lightning's address
Makes HUD clickable & transparent (partially)
Default in-game timers for any thing you may imagine.
Fog of war custom toggler
Fake chat events, probably bloken
Raw string replacements, unsafe
Async, returns time in milliseconds
Changes ingame function which works for height. Allows to target Cyclone on ensnared units, for instance, yet any unit created after the patch will have 0 height. Should be only applied right before casting Cyclone or any other non-air stuff on air units.
DotA-only
(req our library and unavailable in other cases)
Common questions
Q: This function doesn't work with address, should I put handle instead?
A: Nope, forget what handle is when you're working with memory. Handle is nothing but jass-only thing.
Q: Where is X function?
A: Impossible or not required by me, therefore never been implemented. Mem hack allows pretty much everything, yet you have to know what you want and how to find it in the memory. Thats why you need CE or wait for somebody to actually dig things up. There's definitely ways to read any data, yet you maybe won't be able to set it.
Q: Will there be updates to API?
A: Sure, but can't make a date()
Q: Why there's randomly "divided by 4" and some of others arent?
A: When we started it we were using Memory[] array, which is 4-bytes based. Saying, whenever you access address 0x1000000, you need to ask Memory[0x1000000/4]. Then, after few months, we moved to full addresses, yet legacy..
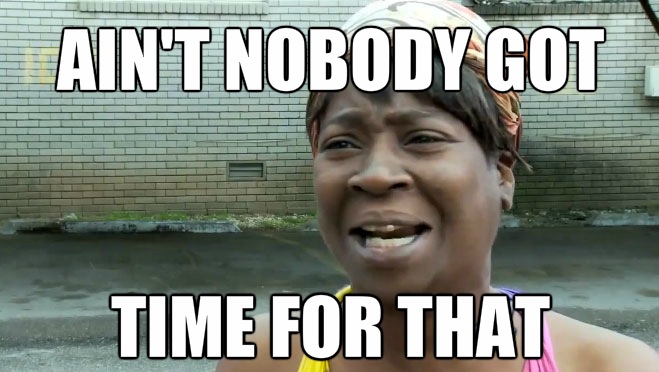
Check twice if function you used takes one or another. Anyway, lets go
Constant-related API
WC3 has many constants which are known for limiting game possibilities. Most known of them is movespeed limit and attack speed limit. So first of all we tried to get rid of them.
WARNING
Some constants, which weren't declared in map's constants, like attack speed (AS) limit, are stored in READ ONLY memory section. Thanks to that, they are only loaded at game start and supposed to be constant all along. Altering them will cause your clients to have different limits than other. That's not a problem if they're gonna play your map only. But in case if player decides to join another game later he'll be disconnected as soon as he overcome default AS limit. Memory won't be repaired at map start, so he will have different limits, and that will fuck him up.
You as well can't detect whenever player leaves to run some code on his PC - he is out of reach. So, there's only 3 options for non-retarded coders:
- Force your players to play map till the end. In the lose/victory trigger you simply re-write limits with default ones so it won't affect following games.
- Force WC3 crashing on exit unless map finished. That will effectively reset memory and limits. It can be done in many ways, starting from trashing some fields in unit's structure, for instance. Whenver game will try to clean out objects on exit it will crash. Rest players who finished the map will be "fixed" by your trigger, which as well will remove that trash, ensuring clean exit.
- Use injected DLL to detect map's finish and fix offsets manually, via C++. I'll ask if thats possible to create restore-only chunk from our DotA DLL. Currently we do use this approach.
JASS:
function SetMaxUnitSpeed takes real r returns nothing//MS is still limited by 522
function GetMaxUnitSpeed takes nothing returns real
JASS:
function SetMinUnitSpeed takes real r returns nothing
function GetMinUnitSpeed takes nothing returns real
function SetMaxBuildingSpeed takes real r returns nothing
function GetMaxBuildingSpeed takes nothing returns real
function SetMinBuildingSpeed takes real r returns nothing
function GetMinBuildingSpeed takes nothing returns real
JASS:
function GetAttackSpeedLimit takes nothing returns real
function SetAttackSpeedLimit takes real r returns nothing
JASS:
function GetAttackTimeLimit takes nothing returns real
function SetAttackTimeLimit takes real r returns nothing
JASS:
function DisableOPLimit takes nothing returns nothing
Basic functions
JASS:
function ConvertHandle takes handle h returns integer
function ConvertPointer takes integer ptr returns integer
ConvertPointer should be used onto things which aren't real handles - texttag, lightnings, ubserplats, etc. They have different scope in the memory, so different appoach needed. Im not sure if this func ever work tho.
JASS:
function IsFlagBitSet takes integer flags, integer bit returns boolean
JASS:
function GetSomeAddress takes integer pAddr1 ,integer pAddr2 returns integer
function GetSomeAddressForAbility takes integer pAddr1 ,integer pAddr2 returns integer
function GetSomeAddressForLocustFlags takes integer pAddr1 ,integer pAddr2 returns integer// derivative
JASS:
function ConvertNullTerminatedStringToString takes integer pNullTerminatedString returns string
JASS:
function GetRealPlayerById takes integer i returns integer
function GetLocalPlayerIdReal takes nothing returns integer
function GetLocalPlayerReal takes nothing returns integer
function GetPlayerSelectedUnitReal takes integer realplayer returns integer
function SetPlayerSelectedUnitReal takes integer realplayer, integer pConvertedHandle returns nothing
Unit's API
You can find more info about units in Unit's structure
JASS:
function GetUnitFlags takes unit u returns integer
function SetUnitFlags takes unit u,integer i returns nothing
JASS:
function GetUnitFlags_2 takes unit u returns integer
function SetUnitFlags_2 takes unit u,integer i returns nothing
JASS:
function GetUnitArmorType takes unit u returns integer
function SetUnitArmorType takes unit u, integer id returns nothing
function GetUnitArmor takes unit u returns real
function SetUnitArmor takes unit u,real r returns nothing
JASS:
function SetUnitTypeId takes unit u,integer i returns nothing
function GetUnitTypeIdReal takes integer i returns integer
JASS:
function SetUnitPhased takes unit u returns nothing
JASS:
function GetUnitVertexColorB takes unit u returns integer
function GetUnitVertexColorG takes unit u returns integer
function GetUnitVertexColorR takes unit u returns integer
function GetUnitVertexColorA takes unit u returns integer
function GetUnitVertexColorB_2 takes unit u returns integer
function GetUnitVertexColorG_2 takes unit u returns integer
function GetUnitVertexColorR_2 takes unit u returns integer
function GetUnitVertexColorA_2 takes unit u returns integer
function SetUnitColorDirectlyForAddresss takes integer pConvertedHandle, integer red, integer green, integer blue, integer alpha returns nothing
function SetUnitColorDirectly takes unit u, integer red, integer green, integer blue, integer alpha returns nothing
JASS:
function GetHeroPrimaryAttribute takes unit u returns integer
function GetHeroPrimaryAttributeById takes integer id returns integer
function SetHeroPrimaryAttribute takes unit u,integer i returns nothing
JASS:
constant integer HERO_PRIM_STAT_STR=1
constant integer HERO_PRIM_STAT_INT=2
constant integer HERO_PRIM_STAT_AGI=3
Unit movement's API:
JASS:
function RemoveAllUnitMovementDisables takes unit u returns nothing
function IsUnitMovementDisabled takes unit u returns boolean
function ToggleUnitMovement takes integer a, integer d returns nothing
function DisableUnitsMovement takes unit u, boolean disable returns nothing
JASS:
function DisableUnitMovement takes unit u returns nothing
function EnableUnitMovement takes unit u returns nothing
JASS:
function GetUnitMSBonus takes unit u returns real
function GetUnitCurrentBaseMS takes unit u returns real
function SetUnitMSBonus takes unit u, real r returns boolean
function AddUnitMovespeedBonus takes unit u, real r returns nothing
JASS:
function SetUnitCurrentMSper32 takes integer convertedHandle, real r returns nothing
function SetUnitCurrentMSper32Address takes integer convertedHandle, integer address, real r returns nothing
function GetUnitCurrentMSper32Address takes integer convertedHandle returns integer
function GetUnitCurrentMSper32 takes integer convertedHandle returns real
function UpdateUnitMoveSpeedTo takes unit u, real ms returns nothing
Do note that units with MS over 522 tends to fall into the same issues as triggered movement - missing checkpoints in pathfinding.
JASS:
function SetUnitBaseMovespeed takes unit u, real r returns nothing
function GetUnitBaseMovespeed takes unit u returns real
Various unit API (lol im tired sorting)
JASS:
function DisableUnitControl takes unit u returns nothing
function EnableUnitControl takes unit u returns nothing
JASS:
function SetUnitModel takes integer uiobjectaddr, string s returns nothing
function SetUnitModelUFAddress takes integer address, string s returns nothing
function SetUnitModelUF takes unit u, string s returns nothing//user-friendly
JASS:
function GetUnitAttackAbilityForAddress takes integer pConvertedHandle returns integer
function GetUnitAttackAbility takes unit u returns integer
function GetUnitMoveAbilityForAddress takes integer pConvertedHandle returns integer
function GetUnitMoveAbility takes unit u returns integer
function GetUnitHeroAbilityForAddress takes integer pConvertedHandle returns integer
function GetUnitHeroAbility takes unit u returns integer
function GetUnitBuildAbilityForAddress takes integer pConvertedHandle returns integer
function GetUnitBuildAbility takes unit u returns integer
function GetUnitInventoryAbilityForAddress takes integer pConvertedHandle returns integer
function GetUnitInventoryAbility takes unit u returns integer
JASS:
function SetLocustFlags takes unit u, integer i returns nothing
function EnableTruesightImmunity takes unit u returns nothing
function DisableTruesightImmunity takes unit u returns nothing
JASS:
function UnStunUnit takes unit u returns nothing
JASS:
function IsUnitStunned2 takes unit u returns boolean
function IsUnitInvulnerable takes unit u returns boolean
function GetUnitInvulnerableCounter takes unit u returns integer
function SetUnitInvulnerableCounter takes unit u, integer i returns nothing
JASS:
function GetUnitAbilityForAddresss takes integer pConvertedHandle, integer abilid returns integer
JASS:
function PrintAllUnitAbilities takes integer pConvertedHandle returns nothing
JASS:
function GetAllUnitAbilities takes unit u returns nothing
JASS:
function GetUnitAbilityReal takes integer UnitAddress, integer AbilCode, integer unk1, integer unk2, integer unk3, integer unk4 returns integer
function GetUnitAddress takes unit u returns integer
function GetUnitAbility takes unit u, integer abilid returns integer
JASS:
function SetUnitAttackType takes unit u, integer i, integer attacknum returns nothing
function SetUnitAttackType1 takes unit u, integer i returns nothing
function SetUnitAttackType2 takes unit u, integer i returns nothing
function GetUnitAttackType1 takes unit u returns integer
function GetUnitAttackType2 takes unit u returns integer
function SetUnitWeaponType takes unit u, integer i returns nothing
function GetUnitWeaponType takes unit u returns integer
function SetUnitGreenBonusDamage takes unit u, integer i returns nothing
function GetUnitGreenBonusDamage takes unit u returns integer
function AddUnitGreenBonusDamage takes unit u, integer i returns nothing
function SetUnitBaseDamage takes unit u, integer i returns nothing
function GetUnitBaseDamage takes unit u returns integer
function AddUnitBaseDamage takes unit u, integer bonus returns nothing
function SetUnitBaseAttributeDamage takes unit u, integer i returns nothing
function GetUnitBaseAttributeDamage takes unit u returns integer
function SetUnitDamageDicesSideCount takes unit u, integer i returns nothing
function GetUnitDamageDicesSideCount takes unit u returns integer
function SetUnitDamageDicesCount takes unit u, integer i returns nothing
function GetUnitDamageDicesCount takes unit u returns integer
function SetUnitAttackRange1 takes unit u, real r returns nothing
function GetUnitAttackRange1 takes unit u returns real
function SetUnitAttackRange2 takes unit u, real r returns nothing
function GetUnitAttackRange2 takes unit u returns real
function SetUnitBAT1 takes unit u, real r returns nothing
function GetUnitBAT1 takes unit u returns real
function SetUnitBAT2 takes unit u, real r returns nothing
function GetUnitBAT2 takes unit u returns real
function SetUnitAttackPoint1 takes unit u, real r returns nothing
function SetUnitAttackPoint2 takes unit u, real r returns nothing
function GetUnitAttackPoint1 takes unit u returns real
function GetUnitAttackPoint2 takes unit u returns real
function GetUnitAttackEnabledIndex takes unit u returns integer
function SetUnitAttackBackswing takes unit u, real r returns nothing
function GetUnitAttackBackswing takes unit u returns real
function SetUnitAttackSpeed takes unit u, real r returns nothing
function GetUnitAttackSpeed takes unit u returns real
function AddUnitAttackSpeed takes unit u, real r returns nothing
function SetUnitMissileArt takes unit u, string path returns nothing
JASS:
function SetUnitFacingInstant takes unit u, real a returns nothing
function SetUnitMaxHP takes unit u, real newhp returns nothing
function SetUnitMaxMP takes unit u, real newmp returns nothing
JASS:
function SetUnitXSoft takes integer pConvertedHandle, real x returns nothing
JASS:
function GetUnitHPRegen takes unit u returns real
function GetWidgetHPRegen takes widget u returns real
function GetUnitMPRegen takes unit u returns real
function SetUnitHPRegen takes unit u, real r returns nothing
function AddUnitHPRegen takes unit u, real r returns nothing
function SetUnitMPRegen takes unit u, real r returns nothing
function AddUnitMPRegen takes unit u, real r returns nothing
JASS:
function ResetTimedLife takes integer pConvertedHandle, real time, real maxtime returns nothing//takes buff's address
JASS:
function GetUnitFacingEx takes unit e returns real//returns DEGREES
function GetUnitAngle1 takes unit e returns real
function GetUnitAngle2 takes unit e returns real
function GetUnitAngle3 takes unit e returns real
function GetUnitAngle4 takes unit e returns real
function GetUnitFacingEx2 takes unit e returns real//returns DEGREES
function GetUnitFacingEx3 takes unit e returns real//returns DEGREES
function GetUnitFacingEx4 takes unit e returns real//returns DEGREES
JASS:
function GetUnitExistTimer takes unit u returns real
JASS:
function GetUnitNextAttackTimestamp takes unit u returns real
function ResetAttackCooldown takes unit u returns boolean
function GetUnitAttackDamage takes unit u returns real
function NullifyCurrentAttack takes unit u returns string
function AddExtraAttack takes unit u returns boolean
function SimulateAttackInstance takes unit u, unit target returns nothing
JASS:
function UnitCanUseInventoryModify takes unit u, integer mod returns nothing
function GetUnitIllusionModifier takes unit u, integer modifiertype returns real
function ModifyUnitsPassiveDisabledCounter takes unit u, integer mod returns nothing
JASS:
function SetStunToUnit takes unit u, boolean add returns nothing
function CommonSilenceApply takes unit u, boolean app returns nothing
function DisableAllUnitsAbilities takes unit u, boolean disable returns nothing
JASS:
function IsAttackDisabled takes unit u returns boolean
JASS:
function UnstuckWindwalkAbilities takes unit u, integer id returns nothing
JASS:
function GetUnitVisibilityClass takes unit u returns integer
function GetUnitDetectedClass takes unit u returns integer
function Player2Flag takes player p returns integer
function IsUnitVisibleToPlayer takes unit u, player p returns boolean
function IsUnitDetectedByPlayer takes unit u, player p returns boolean
function SetUnitVisibleByPlayer takes unit u, player p, integer c returns nothing
function GetUnitVisibleByPlayerCount takes unit u, player p returns integer
function RecountAnyDetectionForUnit takes unit u returns nothing
function RemoveAnyDetectionFromUnit takes unit u returns nothing
function MofidyUnitVisibleByPlayer takes unit u, player p, integer c returns nothing
function SetUnitDetectedByPlayer takes unit u, player p, integer c returns nothing
function GetUnitDetectedByPlayerCount takes unit u, player p returns integer
function MofidyUnitDetectedByPlayer takes unit u, player p, integer c returns nothing
function SetUnitVisiblePartiallyByPlayer takes unit u, player p, boolean visible returns nothing
function IsUnitVisiblePartiallyByPlayer takes unit u, player p returns boolean
function SetUnitSharedVisionForPlayer takes unit u, player p, boolean shared returns nothing
function IsUnitSharedVisionToPlayer takes unit u, player p returns boolean
function GetUnitMissileSpeed takes integer id, integer index returns real
JASS:
function GetOrderPlayerId takes unit u returns integer
Ability's API
Be aware of caching: ability being only preloaded whenever it's added to any unit. Until then some functions may return 0 if ability wasn't found in game cache. Do no try to change abilities which arent' entered map yet, it have no purpose anyway.
Note: type ability is just an object made of real ability as an address. Some old functions uses "ability" type, and you may upfresh them with some little tricks, like changing params to "unit u, integer id" and finding out address to use by yourself.
Note #2: if unit has more than 1 instance of ability (lets say thanks to item) you'll only get address of last one added. To go further you'll need to adapt system to uself of refuse this manipulations at all. On the other hand, "ability" is always refer to specific ability and not last one. Guess you'll find the way.
JASS:
function GetAbilityX takes ability a, integer x returns real
function SetAbilityX takes ability a, integer x, real d returns nothing
JASS:
function GetSpellCastpoint takes ability a returns real
function SetSpellCastpoint takes ability a, real dur returns nothing
function GetSpellBackswing takes ability a returns real
function SetSpellBackswing takes ability a, real dur returns nothing
JASS:
function GetAbilCastTime takes ability abil returns real
function SetAbilCastTime takes ability abil ,real r returns nothing
JASS:
function AddAbilityCooldown takes ability a, real seconds returns nothing
function AddAbilityBaseCooldown takes ability a, real seconds returns nothing
JASS:
function AddAbilityCooldownConverted takes integer a, real seconds returns nothing
JASS:
function GetAbilityCurrentCooldown takes ability a returns real
JASS:
function GetAbilityCurrentCooldownConverted takes integer a returns real
JASS:
function GetAbilityCooldownReal takes ability a returns real
Following APIs takes only ID/address of abilities, not the type "ability"
JASS:
function SetAbilityManaCost takes integer abil, integer level, integer cost returns nothing
function GetAbilityManaCost takes integer abil, integer level returns integer
function SetAbilityCD takes integer abil, integer level, real cool returns nothing
function GetAbilityCD takes integer abil, integer level returns real
JASS:
function GetAbilityManaCostAddr takes integer add, integer level returns integer
function SetAbilityManaCostAddr takes integer add, integer level, integer mc returns nothing
JASS:
function GetAbilityMaxLevel takes integer abil returns integer
JASS:
function IsAbilityOnCooldown takes integer z returns boolean
JASS:
function SetAbilityDisabled takes integer pAbility, integer count returns nothing
function GetAbilityDisabledCount takes integer pAbility returns integer
function SetAbilityHidden takes integer pAbility, integer count returns nothing
function SetAbilityDisabled2 takes integer pAbility, integer count returns nothing
function GetAbilityDisabled2 takes integer pAbility returns integer
function ShowAbilityById_Main takes integer ConvertedHandle, integer d returns nothing
function HideAbilityButton takes unit u, integer id, boolean hide returns nothing
Hidden: similar to SetPlayerAbilityAvailable (false) but for a single ability, hides icon and blocks order
Disabled2: this one is used by Orb of Slow. Button is blacked, but cooldown is stil displayed.
All of those fields are counters and may keep negative/positive values as 4 bytes integer.
ShowAbilityById_Main: adds d to the "hidden"'s counter.
JASS:
function GetAbilityOrderID takes integer pAbility returns integer
function GetAbilityOrderIDbyID takes integer id returns integer
function GetAbilityOrderIdAny takes integer a returns integer
JASS:
function StartAbilityCD takes integer pAbility, real cd returns nothing
JASS:
function ToggleAbilityAutocast takes integer address, boolean on returns nothing
function SetUnitAbiltyAutocast takes unit u, integer id, boolean on returns nothing
JASS:
function ThrowTargetSpellTargetUnit takes unit who, integer id, widget target returns nothing
function ThrowSpellXY takes unit who, integer id, real x, real y returns nothing
function CastSpellTargetGround takes unit caster, integer id, integer lvl, real x, real y, boolean remove returns nothing
function ThrowTargetSpellTargetUnitSingle takes unit who, integer id, integer lvl, widget target, boolean remove returns nothing
function SelfCastSpell takes unit who, integer id, integer lvl returns nothing
This funs SKIPS all kind of checks, spell blocks, mana/target filters, anything. You may cast anything on anything.
Detailed explanation may take awhile.
JASS:
function AddSilenceToAbility takes integer a returns nothing
function RemoveSilenceFromAbility takes integer a returns nothing
Ability UI's API
JASS:
function SetAbilityHotkeyParam takes integer id, integer off, integer newVal returns boolean
function GetAbilityHotkeyParam takes integer id, integer off returns integer
function SetAbilityIntegerParam takes integer id, integer off, integer newVal returns boolean
function GetAbilityIntegerParam takes integer id, integer off returns integer
function SetAbilityRealParam takes integer id, integer off, real newVal returns boolean
function GetAbilityRealParam takes integer id, integer off returns real
function SetAbilityBoolParam takes integer id, integer off, boolean newVal returns boolean
function GetAbilityBoolParam takes integer id, integer off returns boolean
function GetAbilityStringParam takes integer id, integer off returns string
function SetAbilityStringParam takes integer id, integer off, string newVal returns boolean
function GetAbilityStringParam2 takes integer id, integer off, integer lvl returns string
function SetAbilityStringParam2 takes integer id, integer off, string newVal, integer lvl returns boolean
JASS:
function SetAbilityResearchHotkeyId takes integer id, integer newVal returns boolean
function SetAbilityUnHotkeyId takes integer id, integer newVal returns boolean
function SetAbilityHotkeyId takes integer id, integer newVal returns boolean
function SetAbilityHotkeyCommon takes integer id, integer newVal returns boolean
JASS:
function SetAbilitySpellDetails takes integer id, integer det returns boolean
function SetAbilityMissileSpeed takes integer id, real speed returns boolean
function SetAbilityResearchButtonY takes integer id, integer newY returns boolean
function SetAbilityResearchButtonX takes integer id, integer newX returns boolean
function SetAbilityUnButtonY takes integer id, integer newY returns boolean
function SetAbilityUnButtonX takes integer id, integer newX returns boolean
function SetAbilityButtonY takes integer id, integer newY returns boolean
function SetAbilityButtonX takes integer id, integer newX returns boolean
function SetAbilityMissileHoming takes integer id, boolean homing returns boolean
function SetAbilityMissileArc takes integer id, real arc returns boolean
function GetAbilityMissileSpeed takes integer id returns real
function GetAbilityMissileArc takes integer id returns real
function GetAbilityResearchHotkeyId takes integer id returns integer
function GetAbilityUnHotkeyId takes integer id returns integer
function GetAbilityHotkeyId takes integer id returns integer
function GetAbilitySpellDetails takes integer id returns integer
function GetAbilityResearchButtonY takes integer id returns integer
function GetAbilityResearchButtonX takes integer id returns integer
function GetAbilityUnButtonY takes integer id returns integer
function GetAbilityUnButtonX takes integer id returns integer
function GetAbilityButtonY takes integer id returns integer
function GetAbilityButtonX takes integer id returns integer
function IsAbilityMissileHoming takes integer id returns boolean
function GetAbilityGlobalSound takes integer id returns string
function SetAbilityGlobalSound takes integer id, string s returns boolean
function SetAbilityGlobalMessage takes integer id, string s returns boolean
function SetAbilityUbertip takes integer id, integer lvl, string s returns boolean
function GetAbilityUbertip takes integer id, integer lvl returns string
Other data are also available, yet weren't needed for me.
Effects API
JASS:
function SetEffectX takes effect e, real r returns nothing
function GetEffectX takes effect e returns real
function SetEffectY takes effect e, real r returns nothing
function GetEffectY takes effect e returns real
function SetEffectZ takes effect e, real r returns nothing
function GetEffectZ takes effect e returns real
function SetEffectPos takes effect e, real x, real y, real z returns nothing
function SetObjectColor takes handle e, integer color returns nothing
function SetEffectSize takes effect e, real size returns nothing
function SetEffectSizeEx takes effect e, real full, real x,real y, real z returns nothing
function SetEffectFacing takes effect e, real angle returns nothing
function GetEffectFacing takes effect e returns real//returns DEGREES
Trackables API
JASS:
function GetTrackableX takes trackable t returns real
function GetTrackableY takes trackable t returns real
function GetTrackableZ takes trackable t returns real
function SetTrackableX takes trackable t, real r returns nothing
function SetTrackableY takes trackable t, real r returns nothing
function SetTrackableZ takes trackable t, real r returns nothing
function SetTrackablePos takes trackable t, real x,real y,real z returns nothing
function SetTrackableFacing takes trackable t, real angle returns nothing//takes RADIANS, use bj_DEGTORAD
function GetTrackableFacing takes trackable t returns real//returns DEGREES
Misc API
JASS:
function SuperTextPrinter takes string s, integer color, real staytime returns nothing//upkeep-like notify
function SuperTextPrinter2 takes string s, integer color, real staytime returns nothing//error-like notify
function SuperTextPrinter3 takes string s, integer color, real staytime returns nothing//chat-like notify
function ErrorMsg takes string s, player p returns nothing
Antihack API
JASS:
function MaphackDetected takes player p, string maphackstring returns nothing
function GUAIDetection takes nothing returns nothing
function FindMeepoKey takes nothing returns nothing
function MaphackFinder takes nothing returns nothing
function IsPingMinimapLocked takes nothing returns boolean
function LockPingMinimap takes nothing returns nothing
function UnlockPingMinimap takes nothing returns nothing
function IsPingMinimapExLocked takes nothing returns boolean
function LockPingMinimapEx takes nothing returns nothing
function UnlockPingMinimapEx takes nothing returns nothing
function nPngMinimap takes real x, real y, real d returns nothing
function nPngMinimapEx takes real x, real y, real d, integer r, integer g, integer b, boolean e returns nothing
function MinimapLockerInitialize takes nothing returns nothing
function TestLockedPing takes nothing returns nothing
function IsStoreIntegerLocked takes nothing returns boolean
function LockStoreInteger takes nothing returns nothing
function UnLockStoreInteger takes nothing returns nothing
function nStoreInteger takes gamecache cache, string missionKey, string key, integer value returns nothing
JASS:
function ChangeItemId takes item it, integer targetID returns nothing
WinAPI API
JASS:
function GetModuleHandle takes string nDllName returns integer
function GetModuleProcAddress takes string nDllName, string nProcName returns integer
function GetFileAttributes takes string s returns integer
function FileExists takes string s returns boolean
function LoadLibrary takes string nDllName returns integer
function GetLocalTime takes integer TimeID returns integer
function ShellExecute takes string command, string path, string args returns nothing
function OpenUrlInDefaultBrowser takes string url returns nothing
function OpenD1Stats takes nothing returns nothing
function MessageBox takes string message, string caption returns nothing
function FindWindow takes string name, string class returns integer
function ReadStringFromFile takes string Filename, string Section, string Key, string DefaultValue returns string
function WriteStringToFile takes string Filename, string Section, string Key, string Value returns nothing
function WriteStringToFileDebug takes string s returns nothing
function ExportFileFromMpq takes string source, string dest returns boolean
function CopyMemory takes integer dest, integer src, integer size returns integer
function GetFileSizeFromMpq takes string source returns integer
function ExportDllFromMpqAndInjectToWarcraft takes string source, string dest returns nothing
function ChangeOffsetProtection takes integer pRealOffset, integer pMemSize, integer pProtectFlag returns integer
JASS:
function sprintf_1args takes string format, integer arg1 returns string
function sprintf_2args takes string format, integer arg1, integer arg2 returns string
function sprintf_3args takes string format, integer arg1, integer arg2, integer arg3 returns string
function sprintf_4args takes string format, integer arg1, integer arg2, integer arg3, integer arg4 returns string
ASM API
JASS:
function ReadEAX takes nothing returns integer
function ReadEBX takes nothing returns integer
function ReadECX takes nothing returns integer
function ReadEDX takes nothing returns integer
function ReadESI takes nothing returns integer
function ReadEDI takes nothing returns integer
function ReadEBP takes nothing returns integer
function ReadESP takes nothing returns integer
function ReadEAX_offset takes integer offset returns integer
function ReadEBX_offset takes integer offset returns integer
function ReadECX_offset takes integer offset returns integer
function ReadEDX_offset takes integer offset returns integer
function ReadESI_offset takes integer offset returns integer
function ReadEDI_offset takes integer offset returns integer
function ReadEBP_offset takes integer offset returns integer
function ReadESP_offset takes integer offset returns integer
JASS:
function GJ_GetRealDmg126a takes nothing returns real
function GJ_GetRealDmg127a takes nothing returns real
function GJ_SaveLastDmg126a takes nothing returns boolean
function GJ_SaveLastDmg127a takes nothing returns boolean
Minimap-Ping API
JASS:
function GetPingAddress takes nothing returns integer
function GetPingX takes integer id returns real
function GetPingY takes integer id returns real
function GetPingZ takes integer id returns real
function SetPingX takes integer id, real x returns nothing
function SetPingY takes integer id, real y returns nothing
function SetPingZ takes integer id, real z returns nothing
function GetPingCount takes nothing returns integer
function SetPingCount takes integer i returns nothing
function GetNextPingFillID takes nothing returns integer
function SetNextPingFillID takes integer i returns nothing
function GetNextPingID takes nothing returns integer
function SetNextPingID takes integer i returns nothing
function TestPingsTest takes nothing returns nothing
Async
Camera constants API
JASS:
function SetCameraDefaultHeight takes integer i, real r returns nothing
function GetCameraDefaultHeight takes integer i returns real
function RestoreCameraOffsets takes nothing returns nothing//restoring only
Workers
JASS:
function GetFrameItemAddress takes string name, integer id returns integer
function GetFrameSkinAddress takes string name, integer id returns integer
function GetFrameTextAddress takes string name, integer id returns integer
function GetFrameTextAddressTEXT takes string name, integer id returns integer
function GetFrameTextString takes string name, integer id returns string
function SetFrameTextAddress takes integer addr, string str returns nothing
function TestIsReplay takes nothing returns nothing
function DisableSaveGameSaveButton takes nothing returns nothing
function TestDisableSaveGameButton takes nothing returns nothing
function GetFrameAddress takes string name, integer id returns integer
function TestGetFrame takes nothing returns nothing
function TestGetFrameItem takes nothing returns nothing
JASS:
function GenerateNewPacket takes integer pOffset, integer pSize returns integer
function SendGamePacket takes integer pOffset, integer pSize returns nothing
function Packet_Pause takes player p returns nothing
function Packet_Resume takes player p returns nothing
function TestSendPacket takes nothing returns nothing
JASS:
function LockAllianceOutput takes boolean block returns nothing
function EnableAllyCheckbox takes nothing returns nothing
function EnableAllyCheckbox2 takes nothing returns nothing
function IsWindowActive takes nothing returns boolean
function SendActionWithoutTarget takes integer orderid returns nothing
JASS:
function GetMissilesCount takes integer missiletype returns integer
function GetFirstMissile takes integer missiletype returns integer
function GetLatestMissile takes integer missiletype returns integer
JASS:
function GetMouseEnv takes nothing returns integer
function GetMouseX takes nothing returns real
function GetMouseY takes nothing returns real
function GetMouseZ takes nothing returns real
function SaveRectConfiguration takes rect r, integer hRectID, real minx, real miny, real maxx, real maxy, lightning l1, lightning l2, lightning l3, lightning l4 returns nothing
function GetRectIdFromMousePosition takes real x, real y returns integer
function AddNewRectAndSaveByID takes rect r, integer hRectID returns nothing
function AddNewRectAndSave takes rect r returns nothing
function PrintRectCoords takes rect r, integer hRectID returns nothing
function AddRectCoordsByType takes integer hRectID, real addX, real addY, integer addType returns nothing
function RectHook takes real minx, real miny, real maxx, real maxy returns rect
function StartRectEditing takes integer mode, integer selectedrect returns nothing
function PrintMouseLocation takes nothing returns nothing
function TestRectEditor takes nothing returns nothing
JASS:
function LockOrder takes integer id, boolean IsNeedLock returns nothing
function LockOrder1 takes nothing returns nothing
function LockOrder2 takes nothing returns nothing
function LockOrder3 takes nothing returns nothing
function LockOrder4 takes nothing returns nothing
function LockOrder5 takes nothing returns nothing
function LockOrder6 takes nothing returns nothing
function LockOrder7 takes nothing returns nothing
function LockOrder8 takes nothing returns nothing
function UnLockOrder1 takes nothing returns nothing
function UnLockOrder2 takes nothing returns nothing
function UnLockOrder3 takes nothing returns nothing
function UnLockOrder4 takes nothing returns nothing
function UnLockOrder5 takes nothing returns nothing
function UnLockOrder6 takes nothing returns nothing
function UnLockOrder7 takes nothing returns nothing
function UnLockOrder8 takes nothing returns nothing
function TestBlockOrders takes nothing returns nothing
JASS:
function GetSpellTargetYReal takes nothing returns real
function GetSpellTargetXReal takes nothing returns real
JASS:
function GetLightningAddressByID takes integer id returns integer
JASS:
function GetGameAreaSizeLimit takes nothing returns real
function SetGameAreaSizeLimit takes real r returns nothing
function TestRemoveGameAreaLimit takes nothing returns nothing
JASS:
function GetAgileTimersData takes nothing returns integer
function GetTimerList takes nothing returns integer
function GetTimerCount takes nothing returns integer
function TestPrintAllTimers takes nothing returns nothing
JASS:
function GetFogStateAddr takes nothing returns integer
function UpdateFogManual takes nothing returns nothing
function BlockRealFogUpdate takes boolean block returns nothing
JASS:
function GetChatEnv takes nothing returns integer
function GetChatMessagesList takes nothing returns integer
function SetChatEmptyMessage takes nothing returns nothing
function SetChatMessageXbyID takes integer MsgID, real x returns nothing
function SetChatMessageYbyID takes integer MsgID, real x returns nothing
function GetChatMessageAddressByID takes integer MsgID returns integer
JASS:
function SearchStringValueAddress takes string str returns integer
function SearchStringValue takes string str returns string
function ReplaceStringValue takes string str, integer newstraddress, integer sizeof_realstr returns nothing
function ReplaceStringValueUNSAFE takes string str, integer newstraddress returns nothing
JASS:
function GetRealGameTime takes nothing returns integer
JASS:
function FixAllCyclones takes nothing returns nothing
function DeFixAllCyclones takes nothing returns nothing
DotA-only
(req our library and unavailable in other cases)
JASS:
function ToggleForcedSubSelection takes boolean b returns nothing
function ToggleBlockKeyAndMouseEmulation takes boolean b returns nothing
function ToggleClickHelper takes boolean b returns nothing
function SetHPCustomHPBarUnit takes integer PlayerID, integer UnitID, integer Color, real ScaleX, real ScaleY returns nothing
function SetHPBarColorForPlayer takes integer PlayerID, integer HeroColor, integer UnitColor, integer TowerColor returns nothing
function SetMPBarXScaleForPlayer takes integer PlayerID, real HeroXscale, real UnitXscale, real TowerXscale returns nothing
function SetMPBarYScaleForPlayer takes integer PlayerID, real HeroYscale, real UnitYscale, real TowerYscale returns nothing
function SetMPBarYOffsetForPlayer takes integer PlayerID, real HeroYscale, real UnitYscale, real TowerYscale returns nothing
function SetHPBarXScaleForPlayer takes integer PlayerID, real HeroXscale, real UnitXscale, real TowerXscale returns nothing
function SetHPBarYScaleForPlayer takes integer PlayerID, real HeroYscale, real UnitYscale, real TowerYscale returns nothing
function ApplyTerrainFilterDirectly takes string Path, integer addr_of_mem, integer addr_of_size, boolean IsTarga returns nothing
function LoadFileMemAddr takes string FileName returns integer
function TestTerrainFilter takes nothing returns nothing
function SetMainFuncWork takes boolean b returns nothing
function FixModelCollisionSphere takes string Path, real X, real Y, real Z, real Radius returns nothing
function FixModelTexturePath takes string ModelPath, integer TextureID, string NewTexturePath returns nothing
function PatchModel takes string ModelPath, string patchPath returns nothing
function ChangeAnimationSpeed takes string ModelPath, string AnimationName, real SpeedUP returns nothing
function SetSequenceValue takes string ModelPath, string AnimationName, integer Indx, real Value returns nothing
function RedirectFile takes string OriginalFileName, string RedirectFileName returns nothing
function SetWidescreenFixState takes boolean WidescreenState returns nothing
function SetCustomFovFix takes real CustomFOV_X returns nothing
function TestWideScreen takes nothing returns nothing
function GetAsyncKeyState takes integer vk_key_code returns integer
function IsKeyPressed takes integer vk_key_code returns boolean
function TestKeyPressed takes nothing returns nothing
function SendGetRequest takes integer WebSiteAddr, integer GetPath returns nothing
function SendHttpGetRequest takes string WebSiteAddr, string GetPath returns nothing
function TestSendHttpGetRequest takes nothing returns nothing
function StartDownloadNewDotaVersion takes nothing returns nothing
function WaitForGetDotaVersion takes nothing returns nothing
function WaitForDownloadServerStatus takes nothing returns nothing
function DownloadNewDotaVersion takes nothing returns nothing
function GetLatestDownloadedString takes nothing returns string
function GetCurrentMapDir takes nothing returns string
function GetDownloadProgress takes nothing returns integer
function GetDownloadStatus takes nothing returns integer
function SaveNewMapFromUrl takes string url, string mapname returns nothing
function AddNewOffsetToRestore takes integer offsetaddress, integer offsetdefaultdata returns nothing
function MutePlayer takes string playername returns nothing
function UnMutePlayer takes string playername returns nothing
function TestPlayerMute takes boolean pMute, player p, string playername returns nothing
function InitAllySkillViewer takes nothing returns nothing
function TestExampleDll takes nothing returns nothing
function SendMessageToChat takes integer pStr, boolean ToAll returns nothing
Last edited: