- Joined
- Aug 31, 2009
- Messages
- 775
Hello All,
I'm trying to make a spawn system for a kind of swarm survival game, and the spawning trigger spawns enemies in a "donut" shape around each hero, like this:
This means monsters spawn within 1800 and 2400 distance of your hero. (out of your line of sight, but not so far away as to take a long time to reach you.)
However, I want each player to have his own spawning ring, so as the players run around, they may have their ring intersect with another players. If so, this would cause double spawning in the areas they intersect. This is okay though! I want this behaviour.
However, the problems appear when a player moves close enough to another hero that their spawn donut gets extremely close (or on top) of another player. I don't want units to spawn inside the donut of another player. Like this:
There's also one final problem. It's possible to arrange the players in such a way that one player has a fully teal / cyan area, like this:
In the case where a player is totally surrounded, and no valid location can be found, I want it to pick one of the other player's spawn positions and add it to their donut instead.
So far, this is the code I've made.
tl;dr
How do I make spawns in a donut shape around a unit / hero without spawning too close to another unit who also has its own donut, and secondly how do I move the spawn to a nearby other donut if that player is completely surrounded by the "safe zone" of other units.
I'm trying to make a spawn system for a kind of swarm survival game, and the spawning trigger spawns enemies in a "donut" shape around each hero, like this:
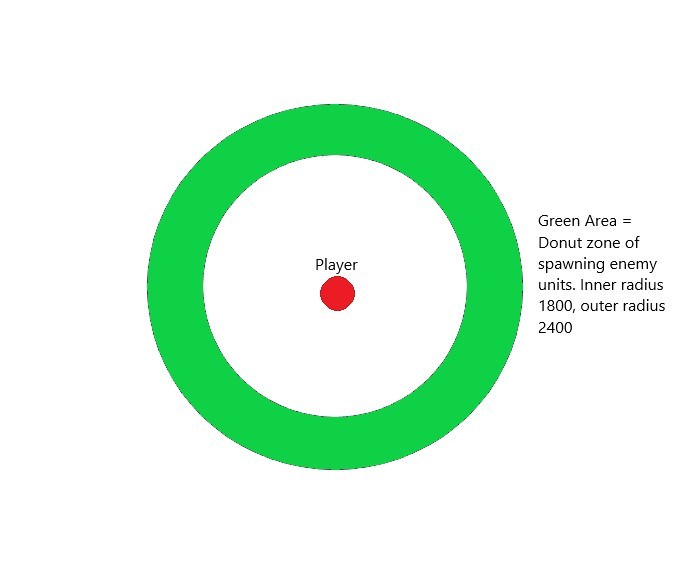
This means monsters spawn within 1800 and 2400 distance of your hero. (out of your line of sight, but not so far away as to take a long time to reach you.)
However, I want each player to have his own spawning ring, so as the players run around, they may have their ring intersect with another players. If so, this would cause double spawning in the areas they intersect. This is okay though! I want this behaviour.
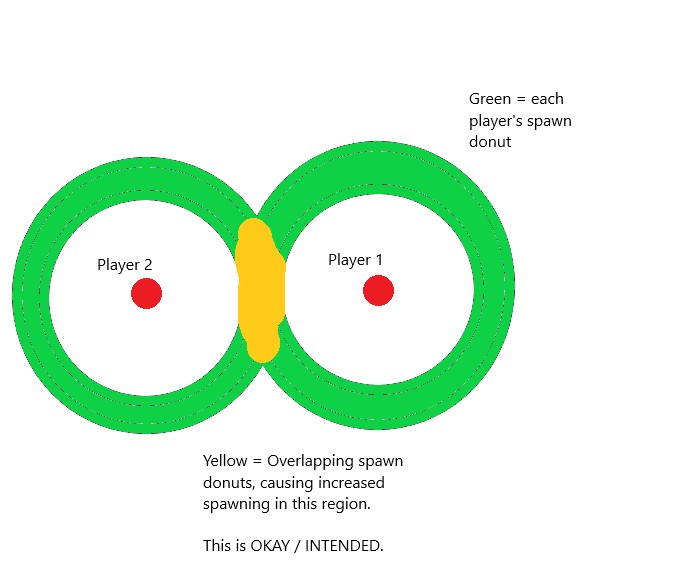
However, the problems appear when a player moves close enough to another hero that their spawn donut gets extremely close (or on top) of another player. I don't want units to spawn inside the donut of another player. Like this:
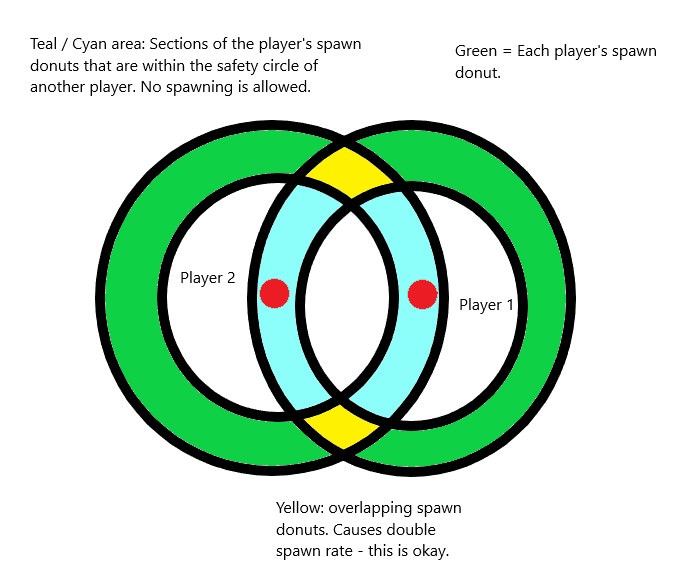
There's also one final problem. It's possible to arrange the players in such a way that one player has a fully teal / cyan area, like this:
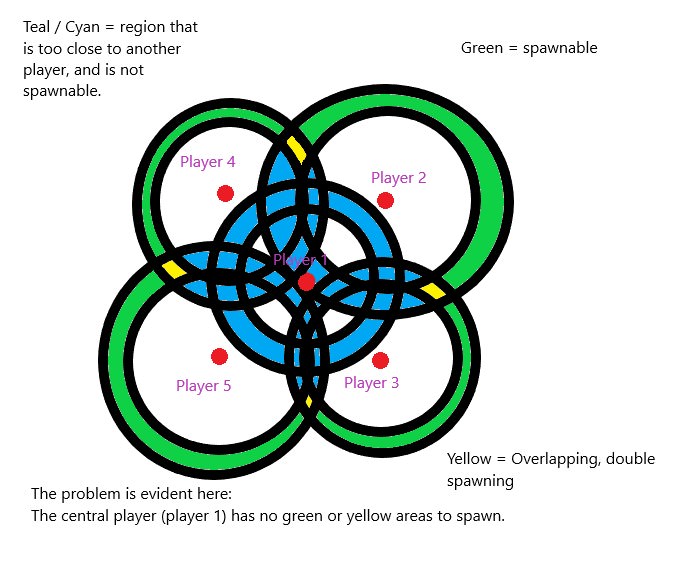
In the case where a player is totally surrounded, and no valid location can be found, I want it to pick one of the other player's spawn positions and add it to their donut instead.
So far, this is the code I've made.
-
Spawn
-
Events
-
Time - Every 0.10 seconds of game time
-
-
Conditions
-
Actions
-
Set temp_group = (Units of type Mercenary)
-
Unit Group - Pick every unit in temp_group and do (Actions)
-
Loop - Actions
-
Set SpawnOrigin = (Position of (Picked unit))
-
Set temp_group2 = (Units within 2600.00 of SpawnOrigin matching (((Owner of (Matching unit)) Equal to Player 23 ($$Dark Green)) and (((Custom value of (Matching unit)) Equal to (Player number of (Owner of (Picked unit)))) and (((Matching unit) is alive) Equal to True))))
-
If (All Conditions are True) then do (Then Actions) else do (Else Actions)
-
If - Conditions
-
(Number of units in temp_group2) Greater than or equal to 40
-
-
Then - Actions
-
Else - Actions
-
Set SpawnFailureCount = 0
-
-------- Find Spawn Location --------
-
Trigger - Run Spawn Location Finder <gen> (ignoring conditions)
-
-------- Define New Intervals --------
-
Unit - DO THE SPAWN STUFF (LOTS OF CODE HERE - ALREADY FINISHED)
-
Custom script: call RemoveLocation(udg_SpawnPoint)
-
-
-
Custom script: call DestroyGroup(udg_temp_group2)
-
Custom script: call RemoveLocation(udg_SpawnOrigin)
-
-
-
Custom script: call DestroyGroup(udg_temp_group)
-
-
-
Spawn Location Finder
-
Events
-
Conditions
-
Actions
-
Set temp_point = (SpawnOrigin offset by (Random real number between 1800.00 and 2400.00) towards (Random angle) degrees)
-
If (All Conditions are True) then do (Then Actions) else do (Else Actions)
-
If - Conditions
-
(Terrain pathing at temp_point of type Walkability is off) Equal to True
-
-
Then - Actions
-
Set SpawnFailureCount = (SpawnFailureCount + 1)
-
Custom script: call RemoveLocation(udg_temp_point)
-
Trigger - Run (This trigger) (ignoring conditions)
-
Skip remaining actions
-
-
Else - Actions
-
-
Set SpawnPoint = (temp_point offset by 0.00 towards 0.00 degrees)
-
Custom script: call RemoveLocation(udg_temp_point)
-
-
tl;dr
How do I make spawns in a donut shape around a unit / hero without spawning too close to another unit who also has its own donut, and secondly how do I move the spawn to a nearby other donut if that player is completely surrounded by the "safe zone" of other units.
Last edited: