Hi, so I've been trying to make a missile system in vJASS, it's still not complete but my problem is, it doesn't create the missile unit.
That's the create method of my missile struct and the other function I use to call it, whenever I create a unit then the "Failed to create unit" message pops up meaning that new.u was null. I don't know what I'm doing wrong. This is how I called the function(From a trigger):
I know the unit name is correct because I created a custom unit with that name:
Anyone can help me with this problem?
JASS:
static method create takes real x, real y, real angle, player owner, string unitname, real speed, real climbrate, real maxdist, unit target, attacktype atktype, damagetype dmgtype, real dmg, real dmgradius, boolean has_deathdmg returns Projectile
local Projectile new = Projectile.allocate()
if maxdist < MIN_MAXDIST then
set maxdist = MIN_MAXDIST
endif
set new.u = CreateUnitByName(owner, unitname, x, y, angle)
set new.target = target
set new.owner = owner
set new.speed = speed
set new.climbrate = climbrate
set new.atktype = atktype
set new.dmgtype = dmgtype
set new.dmgradius = dmgradius
set new.dmg = dmg
set new.has_deathdmg = has_deathdmg
set INDEX_NUM = INDEX_NUM + 1
set TOTAL_PROJ = TOTAL_PROJ + 1
if (new.u == null) then
call BJDebugMsg("Failed to create unit")
else
call BJDebugMsg("Unit created!")
endif
call GroupAddUnit(ProjGroup, new.u)
call SetUnitInvulnerable(new.u, true)
call UnitAddAbility(new.u, 'Aloc')
if INDEX_NUM == 1 then
call TimerStart(ProjTimer, PeriodicTime, true, function ProjectileUpdate)
endif
return new
endmethod
function SpawnProjectile takes real x, real y, real angle, player owner, string unitname, real speed, real climbrate, real maxdist, unit target, attacktype atktype, damagetype dmgtype, real dmg, real dmgradius, boolean has_deathdmg returns nothing
call Projectile.create(x, y, angle, owner, unitname, speed, climbrate, maxdist, target, atktype, dmgtype, dmg, dmgradius, has_deathdmg)
endfunction
|
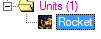
Anyone can help me with this problem?

Last edited by a moderator: